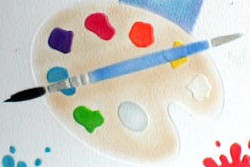
A simplistic definition of a chart is: "an image with several figures of various shapes and color." A custom skin specifies a set of color/styles that is used to "paint" each shape in the chart.
Paint Elements are objects that hold information about color, texture, and other visual styles. Drawing an analogy from an artist’s perspective, a Paint Element is an instance of a paintbrush of a certain shape and size, after it has been dipped in color on the palette.
A skin can be described as:
a set of shapes, and
a set of colored brushes or "paint elements."
A skin allows for the association of these shapes with paint elements. This is done by setting the chart color model to a CustomSkin and adding paint elements to the PaintElement collection of the color model. This can be done using the following code:
In Visual Basic:
UltraChart.ColorModel.ModelStyle = ColorModels.CustomSkin UltraChart.ColorModel.Skin.PEs.Add(new PaintElement(Color.Red)) UltraChart.ColorModel.Skin.PEs.Add(new PaintElement(Color.Green))
In C#:
UltraChart.ColorModel.ModelStyle = ColorModels.CustomSkin; UltraChart.ColorModel.Skin.PEs.Add(new PaintElement(Color.Red)); UltraChart.ColorModel.Skin.PEs.Add(new PaintElement(Color.Green));
The above code sets the color model as a custom skin and adds two paint elements to the custom skin. The following figure shows the concept of coloring the chart with PaintElements. The chart on the left is without a skin, followed to the right by set of vertical gradient paint elements (or PEs), and finally the chart on right is the custom skinned chart.
In the following figure, the chart on the left is without a skin, followed to the right by a set of solid fill color paint elements (or PEs), and finally the chart on right is the custom skinned chart.
Paint elements can be applied to charted items row-wise or column-wise. The individual paint elements can be applied in order across each item (column), or each series (row). The following property can be used to change the way the skin is applied on the charted items:
In Visual Basic:
UltraChart.ColorModel.Skin.ApplyRowWise = true
In C#:
UltraChart.ColorModel.Skin.ApplyRowWise = true;
Primitives and certain ChartAppearance sub-classes that expose properties with sophisticated filling features use PaintElement objects to describe the characteristics of how they are filled and outlined. This Type encapsulates those methods and properties developers may already expect from Brush ( Fill ) and Pen ( Stroke ) objects in other GUI environments. PaintElement objects are only applicable to objects that support all of the following sophisticated filling techniques:
Solid fills
Tiled texture fills
Gradient fills
Hatch pattern fills
ImageFitStyle - Identifies the Fit style applied to Image.
ElementType - Identifies the type of painting applied. A object may actively only be one of the types in the enumeration. Setting a property will automatically update the property with the most current value. It is sometimes convenient for developers to pre-initialize several sets of paint properties (a texture, a gradient and a hatch for instance) and then switch between them by adjusting the PaintElement’s type with one assignment during the application’s processing.
ImageWrapMode - Defines how textures should be tiled and/or wrapped. This property only applies to texture fills specified with the FillImage property. Please see the documentation for WrapMode for further information on tiling of textures.
Hatch - Hatchwork fill pattern applied to the graphical object. Specifies the FillHatchStyle for pattern filling of the associated Primitive or Appearance object.
Fill - Fill color of the associated graphical object. Fill is used interchangeably as the solid fill color or the starting color for gradient fills (see also the FillStopColor property), depending on whether the FillGradientStyle has been set, and the foreground color for FillHatchStyle pattern fills when the Hatch property is set.
FillStopColor - Secondary fill color for the associated graphical object. This property value is only used when the FillGradientStyle or Hatch property has been set to one of the supported gradient or hatch patterns. A gradient is a Bell Curve blend from the Fill color to the FillStopColor. In a hatch, this property describes the background color.
FillStopOpacity -An opacity for the secondary rendering brush. A value of 0 represents a transparent brush, whereas a value of 255 represents a completely opaque brush (items behind the filled area are not visible). Values between 0 and 255 represent varying degrees of translucency. Although it is possible to assign a color with an alpha component to FillStopColor, developers should assign such opacity values using this property instead.
FillOpacity - An opacity for the rendering brush. A value of 0 represents a transparent brush, whereas a value of 255 represents a completely opaque brush (items behind the filled area are not visible). Values between 0 and 255 represent varying degrees of translucency. Although it is possible to assign a color with an alpha component to Fill, developers should assign such opacity values using this property instead.
FillGradientStyle - Determines the gradient fill style applied to the Paint Element’s brush. The gradient fill is a linear interpolation of colors starting from the Fill and running to the FillStopColor, where the change in color is in the direction indicated by the gradient vector.
FillImage - Texture to apply to the graphical object.
Stroke - Color of the pen used to outline drawn shapes. Use this property to affect the color of shape outlines). There are several methods to deactivate the pen used for outlining Primitives when this functionality is not desired, including:
Set this property to Color.Transparent. This is the preferred means of turning off the use of a pen. Alternatively, set the StrokeWidth property to 0 (this is technically equivalent to setting this property to Transparent).
While colors with an alpha component may be assigned directly to Stroke, it is highly recommended that developers use the StrokeOpacity property instead.
StrokeOpacity - An opacity for the rendering pen. A value of 0 represents a transparent pen, whereas a value of 255 represents a completely opaque pen. Values between 0 and 255 represent varying degrees of translucency.
This property value overrides any alpha component of the color defined by Stroke.
StrokeWidth - Thickness of the pen used to outline graphical objects.