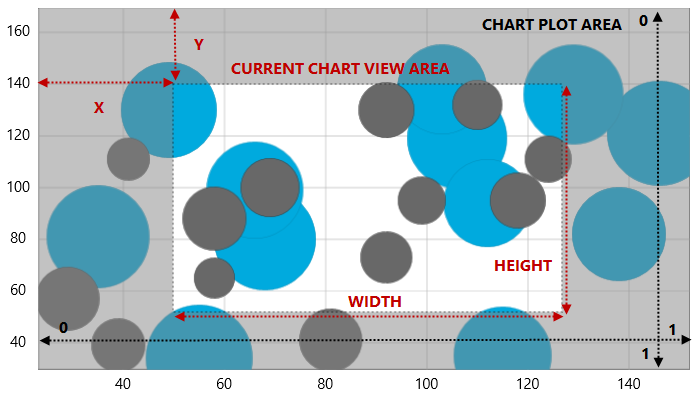
This topic provides information how to navigate content in the UltraDataChart™ control in code-behind.
The following table lists the topics required as a prerequisite to understanding this topic.
This topic contains the following sections
The UltraDataChart control provide several navigation properties that are updated each time a panning or zooming action is performed on the chart. The following table list navigation properties in the chart control:
Figure 1: The UltraDataChart control with the navigation properties’ values visible
Navigation of the UltraDataChart control can be managed from code-behind by changing values of the properties listed in Table 2. The following code snippets demonstrate how to zoom and pan chart from code-behind.
In Visual Basic:
' zoom in by factor of 0.05 Me.DataChart.WindowScaleHorizontal -= 0.05 Me.DataChart.WindowScaleVertical -= 0.05 ' zoom out by factor of 0.05 Me.DataChart.WindowScaleHorizontal += 0.05 Me.DataChart.WindowScaleVertical += 0.05 ' pan up (north direction) by factor of 0.05 Me.DataChart.WindowPositionVertical -= 0.05 ' pan down (south direction) by factor of 0.05 Me.DataChart.WindowPositionVertical += 0.05 ' pan left (west direction) by factor of 0.05 Me.DataChart.WindowPositionHorizontal -= 0.05 ' pan right (east direction) by factor of 0.05 Me.DataChart.WindowPositionHorizontal += 0.05
In C#:
// zoom in by factor of 0.05 this.DataChart.WindowScaleHorizontal -= 0.05; this.DataChart.WindowScaleVertical -= 0.05; // zoom out by factor of 0.05 this.DataChart.WindowScaleHorizontal += 0.05; this.DataChart.WindowScaleVertical += 0.05; // pan up (north direction) by factor of 0.05 this.DataChart.WindowPositionVertical -= 0.05; // pan down (south direction) by factor of 0.05 this.DataChart.WindowPositionVertical += 0.05; // pan left (west direction) by factor of 0.05 this.DataChart.WindowPositionHorizontal -= 0.05; // pan right (east direction) by factor of 0.05 this.DataChart.WindowPositionHorizontal += 0.05;
There are also two methods on the category and numeric axis that you can call in order to programmatically navigate to a specific item or range. These methods are called ScrollIntoView and ScrollRangeIntoView and they will automatically update the chart’s WindowRect property so it centers on the desired item or range.
For the CategoryXAxis and CategoryYAxis, ScrollIntoView takes in the data item of the category you wish to bring into view. ScrollRangeIntoView takes in the minimum and maximum category index that you wish to display. The index correlates with the items in the ItemsSource.
For the CategoryDateTimeXAxis, only the ScrollIntoView method is available but it accepts a minimum and maximum date range that you want to bring into view.
For the NumericXAxis and NumericYAxis only ScrollRangeIntoView is available. This method takes in the minimum and maximum axis values that wish to be brought into view.
The following topics provide additional information related to this topic.