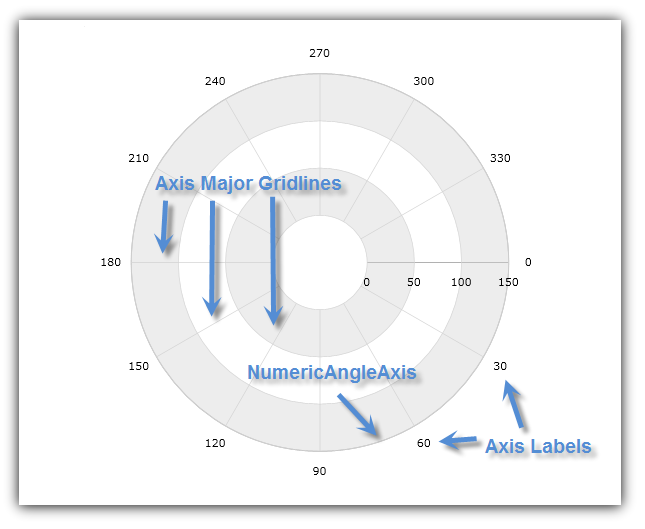
This topic demonstrates, with code examples, how to use Numeric Angle Axis in the UltraDataChart™ control.
The topic is organized as follows:
The NumericAngleAxis has a shape of a circle surrounding the center of chart with major gridlines that look like radial lines starting from the center of the chart and pointing outwards. (Figure 1)
Figure 1: Sample implementation of the NumericAngleAxis shape
This type of axis can be used only with Polar Series (in combination with NumericRadiusAxis) instead of the CategoryAngleAxis (which is used only with Radial Series). For more information on what axis types are required by a specific series, refer to the Series Requirements topic.
The NumericAngleAxis has the following axis crossing properties:
CrossingValue – the radius or distance from the beginning of the NumericRadiusAxis. This value determines the location of intersection of the NumericAngleAxis on the NumericRadiusAxis. Increasing the value of the CrossingValue property will move the NumericAngleAxis farther from the center of the chart and decreasing it will move the angle axis closer to the center along the radius axis. For example, if the NumericRadiusAxis has a range value from 0 to 100 and a value of 50 is set on the CrossingValue property of the NumericAngleAxis then the angle axis will cross the radius axis at value of 50. By default, the crossing value of the angle is set to the maximum value of radius axis, which means that the angle axis will be rendered at the outer ring of the chart
CrossingAxis – the axis in the UltraDataChart control’s Axes collection that crosses the NumericAngleAxis. This property must be bound to a NumericRadiusAxis if there is more than one NumericAngleAxis and one NumericRadiusAxis in the UltraDataChart control’s Axes collection. If you don’t specify the crossing axis, then the angle axis will assume the first axis of the NumericRadiusAxis type in the chart’s Axes collection is the correct crossing axis.
The following code snippet shows how to use the CrossingAxis and CrossingValue properties of the NumericAngleAxis in the UltraDataChart control. It sets the intersection of NumericAngleAxis with NumericRadiusAxis at radius of 150 from the beginning of the NumericRadiusAxis. The result is shown in Figure 2 below.
In Visual Basic:
Dim DataChart As New UltraDataChart() Dim AngleAxis As New NumericAngleAxis() Dim RadiusAxis As New NumericRadiusAxis() RadiusAxis.CrossingAxis = AngleAxis RadiusAxis.CrossingValue = 0 AngleAxis.CrossingAxis = RadiusAxis AngleAxis.CrossingValue = 150 DataChart.Axes.Add(AngleAxis) DataChart.Axes.Add(RadiusAxis)
In C#:
var DataChart = new UltraDataChart(); var AngleAxis = new NumericAngleAxis(); var RadiusAxis = new NumericRadiusAxis(); RadiusAxis.CrossingAxis = AngleAxis; RadiusAxis.CrossingValue = 0; AngleAxis.CrossingAxis = RadiusAxis; AngleAxis.CrossingValue = 150; DataChart.Axes.Add(AngleAxis); DataChart.Axes.Add(RadiusAxis);
Figure 2: The UltraDataChart control with NumericAngleAxis crossing at 150 radius value of NumericRadiusAxis
The NumericAngleAxis has the following range properties:
MinimumValue – the position at which the axis begins
MaximumValue – the position at which the axis ends
Interval – the interval between the consecutive major gridlines or angular separation between radial lines starting from the center of the chart.
By default, the UltraDataChart uses auto range which means that the MinimumValue property will be set to a data point with the smallest angular data column and the MaximumValue property will be set to a data points with the largest angular data column. However, manually setting a value range on the NumericAngleAxis will hide all data points with angular data column outside this range. For example, if a collection of data points with angular data column varying from 0 to 360 is bound to a series and a value range from 50 (MinimumValue) to 100 (MaximumValue) is set on the NumericAngleAxis, then the chart will only show data points with angular data column between 50 and 100.
The following code snippet shows how to use range values with the NumericAngleAxis in the UltraDataChart control. It sets angle range between 0 and 360 and intervals of major gridlines every 30 degrees. The result is shown in Figure 3 below.
In Visual Basic:
Dim DataChart As New UltraDataChart() Dim AngleAxis As New NumericAngleAxis() AngleAxis.MinimumValue = 0 AngleAxis.MaximumValue = 360 AngleAxis.Interval = 50 DataChart.Axes.Add(AngleAxis)
In C#:
var DataChart = new UltraDataChart(); var AngleAxis = new NumericRadiusAxis(); AngleAxis.MinimumValue = 0; AngleAxis.MaximumValue = 360; AngleAxis.Interval = 30; DataChart.Axes.Add(AngleAxis);
Figure 3: NumericAngleAxis with range value between 0 and 260 and interval of 30
The NumericAngleAxis always starts from the 3 o’clock position (the right hand side of the chart). However, this can be changed by setting the NumericAngleAxis object’s StartAngleOffset property to the angle that will be used to offset the starting axis location in a clockwise direction. For example, a value of 90 set on the StartAngleOffset property will position the starting point for the NumericAngleAxis at the 6 o’clock position (the bottom of the chart) and a value of 270 will start this axis at the 12 o’clock position (the top of the chart). The axis index usually increases clockwise, but you can set IsInverted to true on the NumericAngleAxis in order to make the axis indices increase counter-clockwise instead.
The following code snippets show how to offset the starting point of the NumericAngleAxis in the UltraDataChart control by 60 degrees. The result is shown in Figure 4 below.
In Visual Basic:
Dim AngleAxis As New NumericAngleAxis() AngleAxis.StartAngleOffset = 60
In C#:
var AngleAxis = new NumericAngleAxis(); AngleAxis.StartAngleOffset = 60;
Figure 4: NumericAngleAxis with 60 degrees offset for starting angle value