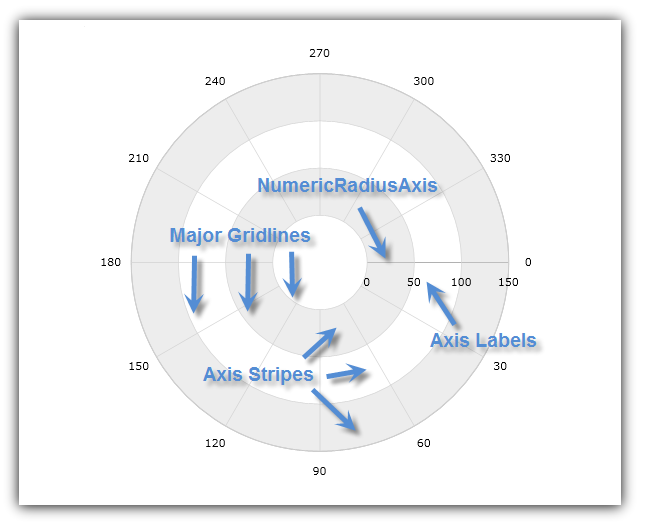
This topic demonstrates, with code examples, how to use Numeric Radius Axis in the UltraDataChart™ control.
The topic is organized as follows:
The NumericRadiusAxis shape of the UltraDataChart™ control is a shape of a straight line starting from center of chart and pointing outwards to the boundary of chart plot area. The major gridlines of this axis look like circles with increasing radius values. (Figure 1)
Figure 1: Sample implementation of the NumericRadiusAxis
shape
This type of axis can be used only with Polar Series (in combination with NumericAngleAxis) or with Radial Series (in combination with CategoryAngleAxis). For more information on what axis types are required by a specific series, refer to the Series Requirements topic.
The NumericRadiusAxis
has the following axis crossing properties:
CrossingValue – the direction to which the NumericRadiusAxis
is pointed. The valid range is from 0 to 360. By the default, this value is set to zero which corresponds to the 3 o’clock position on the CategoryAngleAxis or NumericAngleAxis. The value of the CrossingValue
property indicates the number of degrees with which to shift the NumericRadiusAxis
clockwise from the 3 o’clock position. For example, a value of 90 set on CrossingValue
property orients the NumericRadiusAxis
at the 6 o’clock position and a value of 270 – at the 12 o’clock position.
CrossingAxis – the axis in the UltraDataChart
control’s
Axes
collection that crosses the NumericRadiusAxis
. This property must be bound to a NumericAngleAxis (when using Polar Series) or CategoryAngleAxis
(when using Radial Series), and vice-versa, if there is more than one NumericRadiusAxis
and one NumericAngleAxis
or CategoryAngleAxis
in the Axes
collection of the UltraDataChart control.
Table 1: Axis Types that can be bound to the CrossingAxis
property
The following code snippets show how to use the CrossingAxis
and CrossingValue
properties with NumericRadiusAxis
in the UltraDataChart
control. It sets the intersection of NumericRadiusAxis
with NumericAngleAxis
at angle of zero degrees from the beginning of NumericAngleAxis
. The result is shown in Figure 2 below.
In Visual Basic:
Dim DataChart As New UltraDataChart()
Dim AngleAxis As New NumericAngleAxis()
Dim RadiusAxis As New NumericRadiusAxis()
RadiusAxis.CrossingAxis = AngleAxis
RadiusAxis.CrossingValue = 0
AngleAxis.CrossingAxis = RadiusAxis
AngleAxis.CrossingValue = 150
DataChart.Axes.Add(AngleAxis)
DataChart.Axes.Add(RadiusAxis)
In C#:
var DataChart = new UltraDataChart();
var AngleAxis = new NumericAngleAxis();
var RadiusAxis = new NumericRadiusAxis();
RadiusAxis.CrossingAxis = AngleAxis;
RadiusAxis.CrossingValue = 0;
AngleAxis.CrossingAxis = RadiusAxis;
AngleAxis.CrossingValue = 150;
DataChart.Axes.Add(AngleAxis);
DataChart.Axes.Add(RadiusAxis);
Figure 2: The UltraDataChart control with NumericRadiusAxis
crossing at the 3 o’clock position of NumericAngleAxis
The NumericRadiusAxis
has the following range properties:
MinimumValue – the position at which the axis begins
MaximumValue – the position at which the axis ends
Interval – the interval between the consecutive major gridlines or radius rings of the NumericRadiusAxis
By default, the UltraDataChart
control uses auto range, which means that the MinimumValue
property will be set to the data point with the smallest radius data column and the MaximumValue
property will be set to the data points with the largest radius data column. However, manually setting a value range on NumericRadiusAxis
will hide all data points with radius data column outside this range. For example, if a collection of data points, with a radius data column varying from 0 to 200 bound to a series and a value range of 50 (MinimumValue
) and 100 (MaximumValue
), is set on NumericRadiusAxis
, then the chart will only show data points with radius data column between 50 and 100.
The following code snippets show how to use range values with NumericRadiusAxis
in the UltraDataChart
control. It sets the radius axis range between 0 and 150 and the intervals of the major gridlines to 50. The result is shown in Figure 3 below.
In Visual Basic:
Dim DataChart As New UltraDataChart()
Dim RadiusAxis As New NumericRadiusAxis()
RadiusAxis.MinimumValue = 0
RadiusAxis.MaximumValue = 150
RadiusAxis.Interval = 50
DataChart.Axes.Add(RadiusAxis)
In C#:
var DataChart = new UltraDataChart();
var RadiusAxis = new NumericRadiusAxis();
RadiusAxis.MinimumValue = 0;
RadiusAxis.MaximumValue = 150;
RadiusAxis.Interval = 50;
DataChart.Axes.Add(RadiusAxis);
Figure 3: NumericRadiusAxis
with range value between 0 and 150 and interval of 50
The NumericRadiusAxis
has two unique properties for configuring the axis scale:
RadiusExtentScale – the percentage of the outer radius extent to use as the maximum radius or how far the maximum radius extents from the center of the chart. The valid range is from 0.0 to 1.0.
InnerRadiusExtentScale – the percentage of the inner radius extent to use as the minimum radius or how big blank space should be at the center of the chart. For example, a value of zero set on the InnerRadiusExtentScale
property will result in no blank space in the center of chart. The valid range is from 0.0 to 1.0.
The value for the RadiusExtentScale
property should always be higher than the value for InnerRadiusExtentScale
property in order for the chart to render correctly and display radius lines and axis labels.
The following code snippets show how to use radius extent scales with the NumericRadiusAxis
in the UltraDataChart
control. It increases the inner radius extent to 20% of the chart radius and decreases the outer radius extent to 80% of the chart radius implements. This renders a blank space in the center of the chart and makes space for the labels outside the numerical angle axis. The result is shown in Figure 4 below.
In Visual Basic:
Dim DataChart As New UltraDataChart()
Dim RadiusAxis As New NumericRadiusAxis()
RadiusAxis.RadiusExtentScale = 0.8
RadiusAxis.InnerRadiusExtentScale = 0.2
DataChart.Axes.Add(RadiusAxis)
In C#:
var DataChart = new UltraDataChart();
var RadiusAxis = new NumericRadiusAxis();
RadiusAxis.RadiusExtentScale = 0.8;
RadiusAxis.InnerRadiusExtentScale = 0.2;
DataChart.Axes.Add(RadiusAxis);
Figure 4: The NumericRadiusAxis
with radius scale extenting between 20% and 80% of chart radius