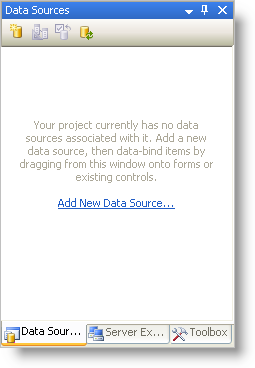
The .NET Language Integrated Query (LINQ) is a querying programming model that can be used with a data source.
You can use LINQ within your programming language, such as C#, to retrieve data and easily manipulate the results. LINQ to SQL is an object relational model implementation that allows you to model a relational database using .NET classes.
This topic will walk you through the process of binding your WinCombo to data using LINQ to SQL.
Before You Begin
Visual Studio 2008 should be installed on your machine.
What You Will Accomplish
This topic will show you how to bind your WinCombo control to data using the LINQ to SQL technology available with Visual Studio 2008.
Follow these Steps
From the toolbox, drag the UltraCombo control to your form.
From the Quick Start Designer, click Finish.
From the Data Sources tab, select Add New Data Source…
The Data Source Configuration Wizard appears.
Select Database, click Next.
In the Choose Your Data Connection dialog box,click New Connection. The Add Connection dialog box appears. To use the LINQ to SQL feature, you must use an SQL Server database.
Change the Data Source to Microsoft SQL Server Database File.
Browse to your SQL Server database.
Click OK.
In the Save the Connection String to the Application Configuration File, click Next.
In the message box that appears, click Yes.
Select the Tables node, then click Finish.
On the Project menu, click Add New Item…
In the Add New Item Dialog box, select LINQ to SQL Classes from the Templates window.
Set Name to NorthWind.dbml
Drag the following item from the Server Explorer on to the design surface:
Product
Category
On the File menu, click Save. This will persist the .NET classes that represent the entities and database relationships that you created.
In the Form1_Load event enter the following code:
In Visual Basic:
'Create an instance of the NorthWindDataContext Dim db As New NorthWindDataContext 'Select all products in the database where the category name is Beverages Dim products = From p In db.Products _ Where p.Category.CategoryName = "Beverages" _ Select New With {.Name = p.ProductName} 'Bind WinCombo to the results Me.UltraCombo1.DataSource = products
In C#:
//Create an instance of the NorthWindDataContext NorthWindDataContext db = new NorthWindDataContext(); //Select all products in the database where the category name is Beverages var products = from p in db.Products where p.Category.CategoryName == "Beverages" select new { Name = p.ProductName }; //Bind WinCombo to the results this.ultraCombo1.DataSource = products;