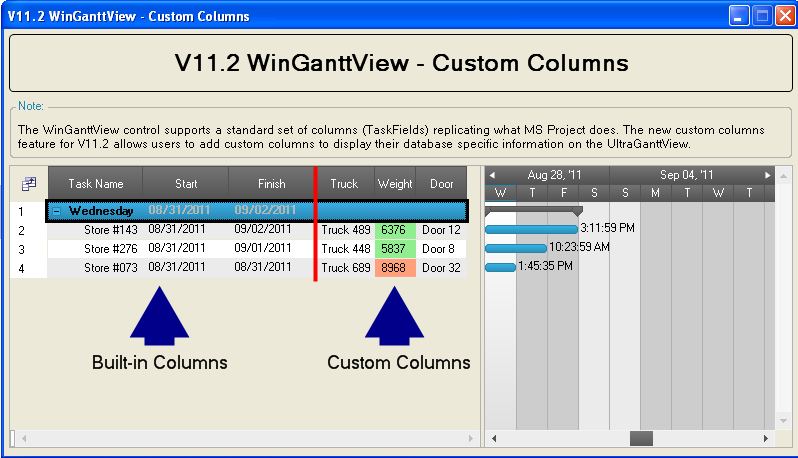
This topic outlines the custom columns support for the WinGanttView™ control and includes code examples to demonstrate usage scenarios.
The topic is organized as follows:
The WinGanttView control supports a standard set of columns (TaskFields) that replicate the Microsft® Project™ defaults. In addition, to these default columns the control also supports custom columns that allow you to tailor your own project specific data fields to display in the WinGanttView.
To add custom columns to the WinGanttView, use the * Add* method found in the * CustomTaskColumns* property of the WinCalendarInfo component. For more information on the configuration and use of WinGanttView, see the topics contained in Using WinGanttView.
Figure 1: WinGanttView custom column sample from the Samples' Browser.
The table below lists the available overloads for the Add method found in the CustomTaskColumns property of the WinCalendarInfo component.
The code below is a snippet from the WinGanttView Custom Columns sample found in the Infragistics samples browser and uses the WinCalendarInfo component instance to add custom columns to the WinGanttView. The examples featured in the code listing create three columns of type string that are not persisted from a data source. For a preview of the custom columns created in this snippet refer to Figure 1 .
In C#:
// Add custom columns calendarInfoShipping.CustomTaskColumns.Add("Truck", typeof(String), false, "Truck 123"); calendarInfoShipping.CustomTaskColumns.Add("Weight", typeof(String), false, "13000"); calendarInfoShipping.CustomTaskColumns.Add("Door", typeof(String), false, "Door 123");
In Visual Basic:
' Add custom columns calendarInfoShipping.CustomTaskColumns.Add("Truck", GetType([String]), False, "Truck 123") calendarInfoShipping.CustomTaskColumns.Add("Weight", GetType([String]), False, "13000") calendarInfoShipping.CustomTaskColumns.Add("Door", GetType([String]), False, "Door 123")
To get or set cell values within the custom columns, you use the * GetCustomProperty* and * SetCustomProperty* methods found in the associated Task.
The code below starts off by setting the cell value of the custom column named Truck in the first WinGanttView task to “Big Truck 113”. The value is then retrieved and casted to the cell value’s data type and placed into the string variable named truckName.
In C#:
// Set a property on a custom column ganttViewShipping.CalendarInfo.Tasks[0].SetCustomProperty("Truck", "Big Truck 113"); // Get a property from a custom column // The GetCustomProperty returns an object, so cast to the proper data type String truckName = (String)ganttViewShipping.CalendarInfo.Tasks[0].GetCustomProperty("Truck");
In Visual Basic:
' Set a property on a custom column ganttViewShipping.CalendarInfo.Tasks(0).SetCustomProperty("Truck", "Big Truck 113") ' Get a property from a custom column ' The GetCustomProperty returns an object, so cast to the proper data type Dim truckName As [String] = DirectCast(ganttViewShipping.CalendarInfo.Tasks(0).GetCustomProperty("Truck"), [String])