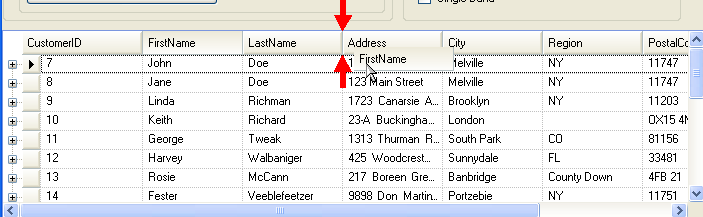
The WinGrid™ has built-in functionality for allowing the user to move and swap columns. By default, column moving is enabled in non-Row Layout mode. In Row Layout mode you must explicitly enable column moving by setting the AllowColMoving to WithinBand. Column swapping is not enabled by default. To enable it, you must set the AllowColSwapping to WithinBand.
You can move a column at run-time by selecting the column, then clicking and dragging it into it’s new position.
Run the program.
Select a Column by clicking on the Column Header. You can click and drag on a column header to select multiple columns.
Once the Column or Columns are selected, click the Column header again and drag the mouse. As you move the mouse over the column headers, you will see red arrow indicating where the columns will be placed when you release the mouse button.
Release the mouse to drop the columns into their new position.
The following snapshot shows column moving in action.
Column swapping allows you to swap the position of two columns. By default, column swapping is disabled.
Before you start writing any code, you should place using/imports directives in your code-behind so you don’t need to always type out a member’s fully qualified name.
In Visual Basic:
Imports Infragistics.Win.UltraWinGrid
In C#:
using Infragistics.Win.UltraWinGrid;
To enable Column Swapping, you must first set the AllowColSwapping property of the Override object. You would typically do this in the InitializeLayout event.
In Visual Basic:
Me.UltraGrid1.DisplayLayout.Override.AllowColSwapping = _
AllowColSwapping.WithinBand
In C#:
this.ultraGrid1.DisplayLayout.Override.AllowColSwapping =
AllowColSwapping.WithinBand;
When the program is run, the Column Header for each row will display a drop-down arrow. To swap columns, drop down the arrow and select the column with which to swap.
As soon as an item is selected from the list, the two columns will switch positions.
The following snapshots shows a swap drop-down. Notice that every column header has a small drop down arrow on the right of it.
If you have column groups defined in the grid, they can be moved and swapped in exactly the same manner as columns. You must set the AllowGroupMoving and AllowGroupSwapping properties as appropriate. Then simply select a group’s header instead of a column’s header, and proceed as outlined above. Note that selecting a group automatically selects all of the columns in that group.
When the user moves or swaps a column via the UI, the BeforeColPosChanged and AfterColPosChanged events fire. Likewise, if the user moves/swaps an UltraGridGroup the BeforeGroupPosChanged and AfterGroupPosChanged fire.
To listen for this interaction, you can trap for when the ColumnPosChangedType is PosChangedType.Moved or Swapped in the event arguments, as demonstrated in the following code:
In C#
private void UltraGrid1_AfterColPosChanged(object sender, AfterColPosChangedEventArgs e)
{
bool hasMovedChanged = (e.ColumnPosChangedType & PosChangedType.Moved) == PosChangedType.Moved;
if(hasMovedChanged)
{
//YOUR CODE HERE
}
}
In VB
Private Sub UltraGrid1_AfterColPosChanged(sender As Object, e As AfterColPosChangedEventArgs)
Dim hasMovedChanged As Boolean = (e.ColumnPosChangedType And PosChangedType.Moved) = PosChangedType.Moved
If hasMovedChanged Then
'YOUR CODE HERE
End If
End Sub