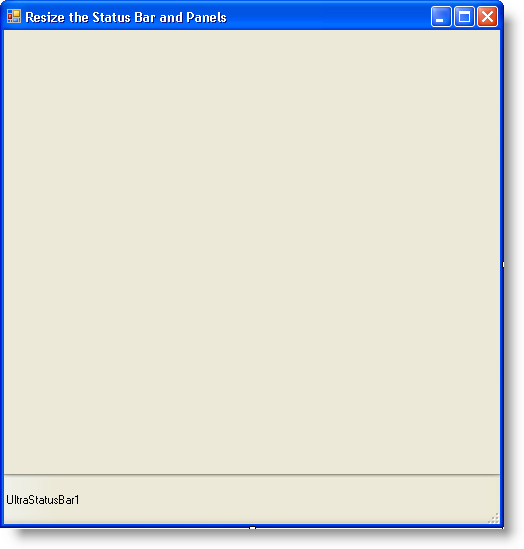
When the SizingMode property of the panel is set to Fixed, which is the default value, or Adjustable, the Width property determines the extent of the panel. The Width property may be set at design time or at run time.
Add an UltraStatusBar to your Windows Form.
In the Property window scroll down to the Panels property. Click the ellipsis to bring up the Panels Collection.
Click the "Add" button. This will add a new panel.
Scroll the properties until you come to the Width property. Changing the width of the panel here will determine it’s width on the status bar.
Click OK to close the window and you will see your panel added to the status bar width the corresponding width.
Scroll to the Size property and expand the plus indicator. There will be 2 properties: Height and Width.
Set the Height property to 50. Use Width to alter the width of the element.
In Visual Basic:
Imports Infragistics.Win.UltraWinStatusBar ... Private Sub Resize_the_Status_Bar_and_Panels_Load(ByVal sender As System.Object, _ ByVal e As System.EventArgs) Handles MyBase.Load ' Create new panel Dim myPanel As New UltraStatusPanel() ' Set the style for the panel myPanel.Style = PanelStyle.Button ' Set the width for the panel myPanel.Width = 200 ' Set some text for the panel myPanel.Text = "New Panel" ' Add the panel to the element Me.UltraStatusBar1.Panels.Add(myPanel) ' Set the height and width of the status bar Dim mySize As New Size(75, Me.Width) Me.UltraStatusBar1.Size = mySize End Sub
In C#:
using Infragistics.Win.UltraWinStatusBar; ... private void Resize_the_Status_Bar_and_Panels_Load(object sender, EventArgs e) { // Create new panel UltraStatusPanel myPanel = new UltraStatusPanel(); // Set the style for the panel myPanel.Style = PanelStyle.Button; // Set the width for the panel myPanel.Width = 200; // Set some text for the panel myPanel.Text = "New Panel"; // Add the panel to the element this.ultraStatusBar1.Panels.Add(myPanel); // Set the height and width of the status bar Size mySize = new Size(75, this.Width); this.ultraStatusBar1.Size = mySize; }