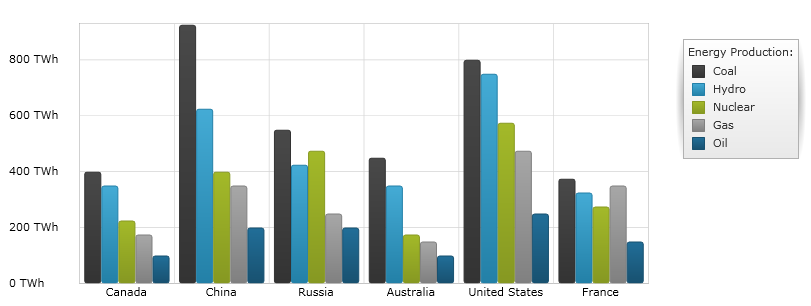
This topic explains, with code example, how to use the ColumnSeries in the XamDataChart™ control.
The topic is organized as follows:
Data Binding
Corners of Column Series
Column Series belongs to a group of Category Series and it is rendered using a collection of rectangles that extend from the bottom to top of the chart towards the values of data points. Column Series emphasizes the amount of change over a period of time or compares multiple items. Values are represented on the y-axis (NumericYAxis) and categories are displayed on the x-axis (CategoryXAxis or CategoryDateTimeXAxis). Column Series uses the same concepts of data plotting as Bar Series but data points are stretched along a horizontal line (x-axis) rather than vertical line (y-axis). In other words, the ColumnSeries is rendered like the BarSeries but with 90 degrees counter-clockwise rotation. For more conceptual information and comprehension with other types of series and supported types of axes, refer to the Category Series and Chart Axes topics.
Figures 1 and 2 demonstrate how the ColumnSeries and the BarSeries look when plotted in the XamDataChart control.
Figure 1: Sample implementation of the ColumnSeries series type.
Figure 2: Sample implementation of the BarSeries series type.
Although the XamDataChart control supports plotting unlimited number of various types of series, it is recommended to use the ColumnSeries with similar types of series. Refer to the Multiple Series topic for information on what types of series are recommended with the ColumnSeries and how to plot multiple types of series.
While the XamDataChart control allows you to easily bind it to your own data model, make sure you supply the appropriate amount and type of data that the series requires. If the data does not meet the minimum requirements based on the type of series that you are using, the control will encounter an error. Refer to the Series Requirements and Category Series topics for more information on data series requirements.
The following is a list of data requirements for the ColumnSeries
type:
The data model must contain at least one numeric property.
The data model may contain an optional string or date time property for labels.
The data source should contain at least one data item.
The ColumnSeries
renders data using the following rules:
Each row in the data column specified as the ValueMemberPath property of the data mapping is drawn as a separate vertical bar.
The string or date time field that is specified as the Label
property of data mapping on the x-axis is used as the category labels. If the Label
token is not specified, default labels are used.
Category labels are drawn on the x-axis. Data values are drawn on the y-axis.
When rendering, multiple series of the ColumnSeries
type that share the same x-axis (see the Multiple Series topic) are rendered in clusters where each cluster represents a data point. The first series in the Series collection of the XamDataChart control renders as a column on the left of the cluster. Each successive series gets rendered on the right of the previous series. However, if series do not share x-axis, they are rendered in layers with each successive series rendered in front of the previous one.
The ColumnSeries
type supports plotting their columns above or below specific reference value using the ReferenceValue property of the y-axis.
The code snippet below shows how to bind the ColumnSeries object to a sample of category data (which is available for download from Sample Energy Data resource). Refer to the data requirements section of this topic for information about data requirements for the ColumnSeries
.
In XAML:
xmlns:local="clr-namespace:Infragistics.Models;assembly=YourAppName"
...
<ig:XamDataChart x:Name="DataChart" >
<ig:XamDataChart.Resources>
<local:EnergyDataSource x:Key="data" />
</ig:XamDataChart.Resources>
<ig:XamDataChart.Axes>
<ig:NumericYAxis x:Name="YAxis" />
<ig:CategoryXAxis x:Name="XAxis" ItemsSource="{StaticResource data}"
Label="{}{Country}" />
</ig:XamDataChart.Axes>
<ig:XamDataChart.Series>
<ig:ColumnSeries ItemsSource="{StaticResource data}" ValueMemberPath="Coal" Title="Coal"
XAxis="{Binding ElementName=XAxis}"
YAxis="{Binding ElementName=YAxis}">
</ig:ColumnSeries>
<ig:ColumnSeries ItemsSource="{StaticResource data}" ValueMemberPath="Hydro" Title="Hydro"
XAxis="{Binding ElementName=XAxis}"
YAxis="{Binding ElementName=YAxis}"
</ig:ColumnSeries>
<ig:ColumnSeries ItemsSource="{StaticResource data}" ValueMemberPath="Nuclear" Title="Nuclear"
XAxis="{Binding ElementName=XAxis}"
YAxis="{Binding ElementName=YAxis}">
</ig:ColumnSeries>
<ig:ColumnSeries ItemsSource="{StaticResource data}" ValueMemberPath="Gas" Title="Gas"
XAxis="{Binding ElementName=XAxis}"
YAxis="{Binding ElementName=YAxis}">
</ig:ColumnSeries>
<ig:ColumnSeries ItemsSource="{StaticResource data}" ValueMemberPath="Oil" Title="Oil"
XAxis="{Binding ElementName=XAxis}"
YAxis="{Binding ElementName=YAxis}">
</ig:ColumnSeries>
</ig:XamDataChart.Series>
</ig:XamDataChart>
In C#:
var data = new EnergyDataSource();
var yAxis = new NumericYAxis();
var xAxis = new CategoryXAxis();
xAxis.ItemsSource = data;
xAxis.Label = "{Country}";
var series = new ColumnSeries();
series.ItemsSource = data;
series.ValueMemberPath = "Coal";
series.Title = "Coal";
series.XAxis = xAxis;
series.YAxis = yAxis;
var chart = new XamDataChart();
chart.Axes.Add(xAxis);
chart.Axes.Add(yAxis);
chart.Series.Add(series);
In Visual Basic:
Dim data As New EnergyDataSource()
Dim yAxis As New NumericYAxis()
Dim xAxis As New CategoryXAxis()
xAxis.ItemsSource = data
xAxis.Label = "Country"
Dim series As New ColumnSeries()
series.ItemsSource = data
series.ValueMemberPath = "Coal"
series.Title = "Coal"
series.XAxis = xAxis
series.YAxis = yAxis
Dim chart As New XamDataChart()
chart.Axes.Add(xAxis)
chart.Axes.Add(yAxis)
chart.Series.Add(series)
Column Series allow setting corners of visual bar elements using the RadiusX and RadiusY properties. Increasing values of these properties will curve the corners of column elements while decreasing the value makes columns more rectangular. The following code snippet demonstrates how to set round corners on the ColumnSeries.
In XAML:
<ig:ColumnSeries RadiusX="10" RadiusY="10" />
In C#:
var series = new ColumnSeries();
series.RadiusX = 10;
series.RadiusY = 10;
...
DataChart.Series.Add(series);
In Visual Basic:
Dim series As New ColumnSeries()
series.RadiusX = 10
series.RadiusY = 10
...
chart.Series.Add(series)
Figure 3: The ColumnSeries with round corners.
Figure 4: The ColumnSeries with rectangular corners.