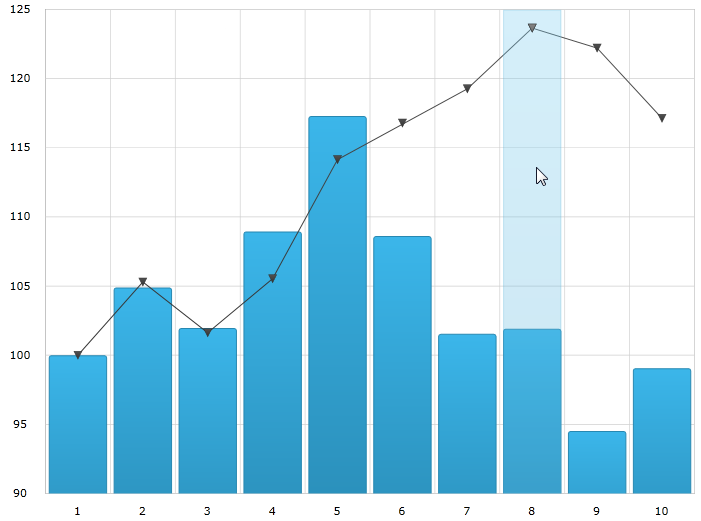
This topic provides information about the category item highlight layer used for hover interactions. It describes the properties of the category item highlight layer and provides an example of its implementation.
The following topics are prerequisites to understanding this topic:
This topic contains the following sections:
The CategoryItemHighlightLayer highlights items in series using category axis by either drawing a banded shape or rendering a marker at that position.
The default highlight depends on the type of series. For example, the highlight for a ColumnSeries and a LineSeries is different; for ColumnSeries it is a banded shape, for LineSeries it is a marker. You can override the default highlight by setting the HighlightType property. For more information on this property, see the Properties section below.
The following image is a preview of the XamDataChart control rendered with the CategoryItemHighlightLayer added.
The following table summarizes the properties of the CategoryItemHighlightLayer.
The following screenshot illustrates how the XamDataChart control with the CategoryItemHighlightLayer object’s
BandHighlightWidth and Brush properties looks as a result of the following setting:
Following is the code used to implement this example
In XAML:
<ig:XamDataChart x:Name="theChart" Margin="5,0,5,0" >
<ig:XamDataChart.Axes>
<ig:CategoryXAxis x:Name="xmCategoryXAxis"
ItemsSource="{StaticResource CategoryData1}"
Label="{}{Category}">
<ig:CategoryXAxis.LabelSettings>
<ig:AxisLabelSettings x:Name="xmCategoryXAxisLabel"
Extent="25"
VerticalAlignment="Bottom"
FontSize="11" />
</ig:CategoryXAxis.LabelSettings>
</ig:CategoryXAxis>
<ig:NumericYAxis x:Name="xmNumericYAxis1">
<ig:NumericYAxis.LabelSettings>
<ig:AxisLabelSettings x:Name="xmNumericYAxisLabel"
Extent="50"
Location="OutsideLeft" />
</ig:NumericYAxis.LabelSettings>
</ig:NumericYAxis>
</ig:XamDataChart.Axes>
<ig:XamDataChart.Series>
<ig:ColumnSeries ItemsSource="{StaticResource CategoryData1}"
ValueMemberPath="Value"
XAxis="{Binding ElementName=xmCategoryXAxis}"
YAxis="{Binding ElementName=xmNumericYAxis1}">
</ig:ColumnSeries>
<ig:LineSeries ItemsSource="{StaticResource CategoryData2}"
ValueMemberPath="Value"
XAxis="{Binding ElementName=xmCategoryXAxis}"
YAxis="{Binding ElementName=xmNumericYAxis1}">
</ig:LineSeries>
<ig:CategoryItemHighlightLayer HighlightType="Marker" Brush="Red" />
</ig:XamDataChart.Series>
</ig:XamDataChart>
In C#:
var catItemHighlightLayerSeries = new CategoryItemHighlightLayer(); catItemHighlightLayerSeries.HighlightType = CategoryItemHighlightType.Marker; catItemHighlightLayerSeries.Brush = new SolidColorBrush(Colors.Red); theChart.Series.Add(catItemHighlightLayerSeries);
In VB:
Dim catItemHighlightLayerSeries As New CategoryItemHighlightLayer() catItemHighlightLayerSeries.HighlightType = CategoryItemHighlightType.Marker; catItemHighlightLayerSeries.Brush = New SolidColorBrush(Colors.Red) theChart.Series.Add(catItemHighlightLayerSeries)