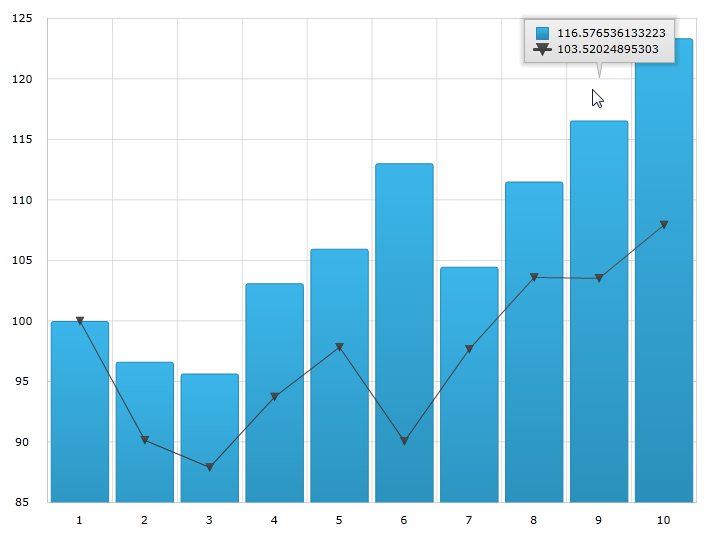
This topic provides information about the category tooltip layer used for hover interactions. It describes the properties of the category tooltip layer and provides an example of its implementation.
The following topics are prerequisites to understanding this topic:
This topic contains the following sections:
The CategoryToolTipLayer displays grouped tooltips for the series on the XamDataChart control using a category axis.
You can configure tooltips to target a specific axis. This can be done be setting the TargetAxis property. For more information on this property, see the Properties section below.
By default the grouped tooltips appear at the top of the XamDataChart control; however this default value can be overridden by setting the ToolTipPosition property. For more information on this property, see the CategoryToolTipLayer properties section below.
The following image is a preview of the XamDataChart control renders with the CategoryToolTipLayer added.
The following table summarizes the properties of the CategoryToolTipLayer layer.
The following screenshot illustrates how the XamDataChart control renders with the CategoryToolTipLayer object’s ToolTipPosition property configured with the following setting:
Following is the code used in this implementation
In XAML:
<ig:XamDataChart x:Name="theChart" Margin="5,0,5,0" >
<ig:XamDataChart.Axes>
<ig:CategoryXAxis x:Name="xmCategoryXAxis"
ItemsSource="{StaticResource CategoryData1}"
Label="{}{Category}">
<ig:CategoryXAxis.LabelSettings>
<ig:AxisLabelSettings x:Name="xmCategoryXAxisLabel"
Extent="25"
VerticalAlignment="Bottom"
FontSize="11" />
</ig:CategoryXAxis.LabelSettings>
</ig:CategoryXAxis>
<ig:NumericYAxis x:Name="xmNumericYAxis1">
<ig:NumericYAxis.LabelSettings>
<ig:AxisLabelSettings x:Name="xmNumericYAxisLabel"
Extent="50"
Location="OutsideLeft" />
</ig:NumericYAxis.LabelSettings>
</ig:NumericYAxis>
</ig:XamDataChart.Axes>
<ig:XamDataChart.Series>
<ig:ColumnSeries ItemsSource="{StaticResource CategoryData1}"
ValueMemberPath="Value"
XAxis="{Binding ElementName=xmCategoryXAxis}"
YAxis="{Binding ElementName=xmNumericYAxis1}">
<ig:ColumnSeries.ToolTip>
<TextBlock Text="{Binding Item.Value}" />
</ig:ColumnSeries.ToolTip>
</ig:ColumnSeries>
<ig:LineSeries ItemsSource="{StaticResource CategoryData2}"
ValueMemberPath="Value"
XAxis="{Binding ElementName=xmCategoryXAxis}"
YAxis="{Binding ElementName=xmNumericYAxis1}">
<ig:LineSeries.ToolTip>
<TextBlock Text="{Binding Item.Value}" />
</ig:LineSeries.ToolTip>
</ig:LineSeries>
<ig:CategoryToolTipLayer ToolTipPosition="InsideStart"
TransitionDuration="0:00:00.1"/>
</ig:XamDataChart.Series>
</ig:XamDataChart>
In C#:
var catToolTipLayerSeries = new CategoryToolTipLayer(); catToolTipLayerSeries.ToolTipPosition = CategoryTooltipLayerPosition.InsideStart; chart.Series.Add(catToolTipLayerSeries);