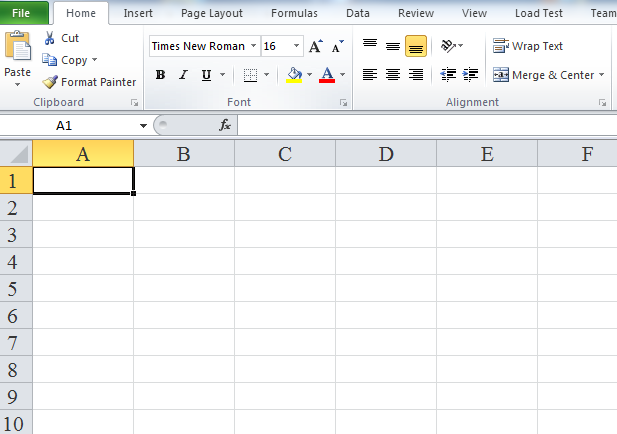
This topic describes how to change the default font of a worksheet when exporting to Microsoft Excel®.
This topic contains the following sections:
There is a special type of style in Infragistics Excel workbook named Normal style, which can be accessed by Workbook.Styles
NormalStyle property. This style contains the default properties for all cells in the workbook, unless otherwise specified on a row, column, or cell. Changing properties of the Normal style will change the default cell format properties in the workbook.
You can change the default font by setting the Workbook.Styles.NormalStyles.StyleFormat
Font property or some of its properties to the desired values.
The code example below creates a new Workbook and adds one sheet to the workbook. It creates a new Font, which will be the default style for the workbook. Font family is changed to Times New Roman and font size is changed to 16 pt. Finally, the workbook is saved.
The following screenshot is a preview of the final result.
As you can see, the default Font family is Times New Roman and the default Font size is 16 pt.
In Visual Basic:
' Create new workbook and add worksheet
Dim workbook As New Infragistics.Documents.Excel.Workbook(Infragistics.Documents.Excel.WorkbookFormat.Excel2007)
Dim worksheet As Infragistics.Documents.Excel.Worksheet = workbook.Worksheets.Add("Sheet1")
Dim normalFont As Infragistics.Documents.Excel.IWorkbookFont = workbook.Styles.NormalStyle.StyleFormat.Font
' Specifying the name of default font
normalFont.Name = "Times New Roman"
' Specifying the font size. Font size height is measured in twips. One twips is 1/20th of a point
normalFont.Height = 16 * 20
Dim saveFileDialog As New SaveFileDialog() With { _
.Filter = "Excel 2007 files|*.xlsx", _
.DefaultExt = "xlsx" _
}
If saveFileDialog.ShowDialog() = True Then
Dim stream As Stream = saveFileDialog.OpenFile()
workbook.Save(stream)
stream.Close()
End If
In C#:
// Create new workbook and add worksheet
Infragistics.Documents.Excel.Workbook workbook = new Infragistics.Documents.Excel.Workbook(Infragistics.Documents.Excel.WorkbookFormat.Excel2007);
Infragistics.Documents.Excel.Worksheet worksheet = workbook.Worksheets.Add("Sheet1");
Infragistics.Documents.Excel.IWorkbookFont normalFont = workbook.Styles.NormalStyle.StyleFormat.Font;
// Specifying the name of default font
normalFont.Name = "Times New Roman";
// Specifying the font size. Font size height is measured in twips. One twips is 1/20th of a point
normalFont.Height = 16 * 20;
SaveFileDialog saveFileDialog = new SaveFileDialog
{
Filter = "Excel 2007 files|*.xlsx",
DefaultExt = "xlsx"
};
if (saveFileDialog.ShowDialog() == true)
{
Stream stream = saveFileDialog.OpenFile();
workbook.Save(stream);
stream.Close();
}
The following topics provide additional information related to this topic.