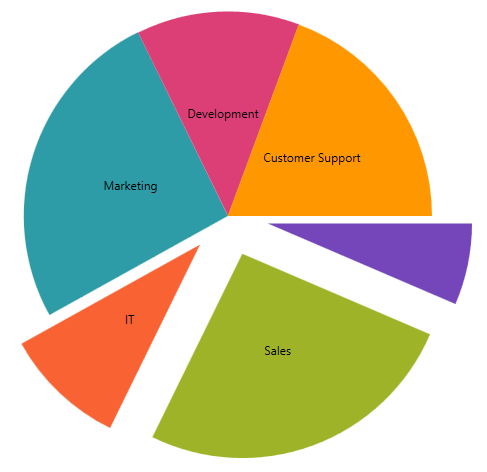
This topic demonstrates how to implement the explosion behavior of the XamPieChart™ control. At the end of the topic, a complete code sample is provided.
The topic is organized as follows:
The XamPieChart control supports explosion of individual pie slices as well as a SliceClick event that allows you to modify selection states and implement custom logic.
Figure 1: The XamPieChart control as implemented by the sample code
This article assumes you have already read the Data Binding topic, and uses the code therein as a starting point.
Configuring the respective properties and event handler
Implementing the event handler
(Optional) Verifying the result
Configure the respective properties and event handler .
Taking code from the Data Binding topic as a starting point, enable explosion by setting the AllowSliceExplosion property to True and configure pieChart_SliceClick as the event handler for mouse clicks:
In XAML:
<ig:XamPieChart Name="pieChart" AllowSliceExplosion="True" SliceClick="pieChart_SliceClick" />
Implement the event handler .
On SliceClick, toggle the explosion states of the slice.
In C#:
private void pieChart_SliceClick(object sender, Infragistics.Controls.Charts.SliceClickEventArgs e) { e.IsExploded = !e.IsExploded; }
In Visual Basic:
Private Sub pieChart_SliceClick(sender As Object, e As Infragistics.Controls.Charts.SliceClickEventArgs) e.IsExploded = Not e.IsExploded Next End Sub
(Optional) Verify the result .
To verify the result, run your application. The Pie Chart control will now respond to SliceClick events by selecting and exploding the appropriate slice outward. A list of currently selected slices will also be maintained in the upper left corner. (Figure 1, above)
The following code listings contain the full example implemented in context.
<UserControl x:Class="XamPieChart_SelectAndExplode.MainPage" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:ig="http://schemas.infragistics.com/xaml" xmlns:ig="clr-namespace:Infragistics.Controls.Charts;assembly=InfragisticsWPF.Controls.Charts.XamDataChart" xmlns:local="clr-namespace:XamPieChart_PieOfPie" mc:Ignorable="d" d:DesignHeight="300" d:DesignWidth="400"> <Grid x:Name="LayoutRoot" Background="White"> <Grid.Resources> <local:Data x:Key="data" /> </Grid.Resources> <ig:ItemLegend Name="Legend" VerticalAlignment="Top" HorizontalAlignment="Right" Margin="10" Padding="10,5,10,5" /> <ig:XamPieChart Name="pieChart" ItemsSource="{StaticResource data}" LabelMemberPath="Label" ValueMemberPath="Value" ToolTip="{}{Label}" LabelsPosition="BestFit" Legend="{Binding ElementName=Legend}" AllowSliceExplosion="True" SliceClick="pieChart_SliceClick" /> </Grid> </UserControl>
In C#:
using System; using System.Collections.ObjectModel; using System.Windows.Controls; namespace XamPieChart_SelectAndExplode { public partial class MainPage : UserControl { public MainPage() { InitializeComponent(); } private void pieChart_SliceClick(object sender, Infragistics.Controls.Charts.SliceClickEventArgs e) { e.IsExploded = !e.IsExploded; } } public class DataItem { public string Label { get; set; } public double Value { get; set; } } public class Data : ObservableCollection<DataItem> { public Data() { Add(new DataItem { Label = "Administration", Value = 20 }); Add(new DataItem { Label = "Sales", Value = 80 }); Add(new DataItem { Label = "IT", Value = 30 }); Add(new DataItem { Label = "Marketing", Value = 80 }); Add(new DataItem { Label = "Development", Value = 40 }); Add(new DataItem { Label = "Customer Support", Value = 60 }); } } }
In Visual Basic:
Imports System Imports System.Collections.ObjectModel Imports System.Windows.Controls Namespace XamPieChart_SelectAndExplode Public Partial Class MainPage Inherits UserControl Public Sub New() InitializeComponent() End Sub Private Sub pieChart_SliceClick(sender As Object, e As Infragistics.Controls.Charts.SliceClickEventArgs) e.IsExploded = Not e.IsExploded End Sub End Class Public Class DataItem Public Property Label() As String Get Return _Label End Get Set _Label = Value End Set End Property Private _Label As String Public Property Value() As Double Get Return _Value End Get Set _Value = Value End Set End Property Private _Value As Double End Class Public Class Data Inherits ObservableCollection(Of DataItem) Public Sub New() Add(New DataItem() With { _ .Label = "Administration", _ .Value = 20 _ }) Add(New DataItem() With { _ .Label = "Sales", _ .Value = 80 _ }) Add(New DataItem() With { _ .Label = "IT", _ .Value = 30 _ }) Add(New DataItem() With { _ .Label = "Marketing", _ .Value = 80 _ }) Add(New DataItem() With { _ .Label = "Developement", _ .Value = 40 _ }) Add(New DataItem() With { _ .Label = "Customer Support", _ .Value = 60 _ }) End Sub End Class End Namespace