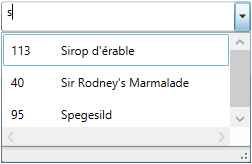
This topic describes how to configure items filtering in the xamComboEditor™ control.
The following topic is a prerequisite to understanding this topic:
This topic contains the following sections:
The following table briefly explains the configurable aspects of the xamComboEditor filtering feature and maps them to the properties that configure them. Further details are available after the table.
The xamComboEditor provides filtering on the items in the control’s Drop-Down when the user starts to type in the input text field. The data items are filtered according to the filters specified in the xamComboEditor .
By default, this feature is enabled, and filtering executed on the data model property specified through the xamComboEditor DisplayMemberPath property and using the comparison operator StartsWith .
The following table maps the desired configuration to the property settings that manage it.
The xamComboEditor control provides functionality for customizing the item filtering.
This is achieved by using the xamComboEditor ItemFilters property. An ObservableCollection
of ComboItemFilter objects is set to this property to provide custom items filtering.
The following table maps the desired configuration to the property settings that manage it.
The following screenshots demonstrate how the filtering in the xamComboEditor works as a result of the following code:
Typing 's' in the xamComboEditor text input field:
Typing '100' in the xamComboEditor text input field:
Following is the code that implements this example.
In XAML:
<ig:XamComboEditor x:Name="ComboEditor"
ItemsSource="{Binding Path=Products}"
Height="30" Width="250"
AutoComplete="False">
<ig:XamComboEditor.ItemTemplate>
<DataTemplate>
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="50" />
<ColumnDefinition />
</Grid.ColumnDefinitions>
<TextBlock Text="{Binding ProductName}" Grid.Column="1" Margin="5" />
<TextBlock Text="{Binding UnitsInStock}" Margin="5" />
</Grid>
</DataTemplate>
</ig:XamComboEditor.ItemTemplate>
<!-- Add custom filters -->
<ig:XamComboEditor.ItemFilters>
<!-- Create filter for the data model property ProductName -->
<ig:ComboItemFilter FieldName="ProductName" LogicalOperator="And">
<ig:ComboItemFilter.Conditions>
<ig:ComparisonCondition Operator="Contains" />
<ig:ComparisonCondition Operator="StartsWith"/>
</ig:ComboItemFilter.Conditions>
</ig:ComboItemFilter>
<!-- Create filter for the data model property UnitsInStock -->
<ig:ComboItemFilter FieldName="UnitsInStock">
<ig:ComboItemFilter.Conditions>
<ig:ComparisonCondition Operator="GreaterThan"/>
</ig:ComboItemFilter.Conditions>
</ig:ComboItemFilter>
</ig:XamComboEditor.ItemFilters>
</ig:XamComboEditor>
The following topics provide additional information related to this topic.