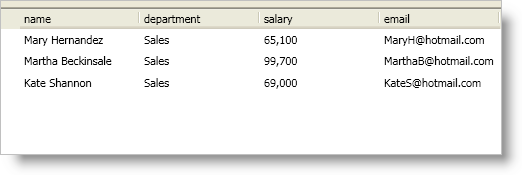
To allow the user to filter records you must set the AllowRecordFiltering property of the FieldSettings object. Even if you did not allowed the user to perform filtering operations you can still programmatically filter records by adding RecordFilter objects to a FieldLayout object’s RecordFilters collection or to a RecordManager object’s RecordFilters collection. You can even programmatically filter records on the xamDataCarousel™ control even though the control does not expose a way for the user to provide record filters.
When you declaratively set the FieldSettings
object’s AllowRecordFiltering
property to true
the control will auto-generate RecordFilter
object for each field in the field layout. These RecordFilter
objects will not be removed from the FieldLayout.RecordFilters
even after invoking the RecordFilters
's Clear
method. However, you can clear their filtering Conditions.
Also as an alternative to adding your own RecordFilter
(s) when programmatically creating record filters, you may use the auto-generated RecordFilter
(s) and modify their filtering Conditions
accordingly.
The RecordFilter
object represents a collection of conditions for the cell values in a field. You can define conditions by adding objects that implement the ICondition
interface to the RecordFilter
object’s Conditions
collection. The Infragistics.Windows.Controls namespace in the InfragisticsWPF.dll assembly offers the following condition objects:
ComparisonCondition - A simple condition that compares values using a comparison operator.
ComplementCondition - A condition that negates an existing condition.
ConditionGroup - A combination of conditions using a logic operator such as 'And' or 'Or'.
Finally, in order for the record filters to affect your records, you must add the RecordFilter
objects to the appropriate RecordFilters
collection. For root-level records, you must add RecordFilter
objects to the FieldLayout
object’s RecordFilters
collection regardless of the RecordFilterScope property value. For child records, you must add the RecordFilter
objects to the appropriate RecordFilters
collection based on the RecordFilterScope
property value of the FieldLayoutSettings object.
For example, if you are binding xamDataPresenter to a hierarchical collection of customers/orders and you are filtering the customer items, you must add RecordFilter
objects to the RecordFilters
collection of the FieldLayout
object that represents the customer class (first FieldLayout
object in the FieldLayouts
collection). If you are filtering the order items and you are using the default RecordFilterScope
property value (SiblingDataRecords), you must add RecordFilter
objects to a child RecordManager
object’s RecordFilters
collection. You can access a child record manager using an ExpandableFieldRecord object’s ChildRecordManager property. On the other hand, if you set the RecordFilterScope
property to AllRecords
, you must add RecordFilter
objects to the RecordFilters
collection of the FieldLayout
object that represents the order items (second FieldLayout
object in the FieldLayouts
collection).
The following example code demonstrates how to add filter conditions.
In XAML:
<!-- You will need the following XML namespace definition to reference the ComparisonCondition object in the Infragistics.Windows.Controls namespace: xmlns:igWindows="http://infragistics.com/Windows" --> <igDP:XamDataPresenter Name="xamDataPresenter1" BindToSampleData="True"> <igDP:XamDataPresenter.FieldLayouts> <igDP:FieldLayout> <igDP:FieldLayout.RecordFilters> <igDP:RecordFilter FieldName="department"> <igDP:RecordFilter.Conditions> <igWindows:ComparisonCondition Operator="Contains" Value="Sales" /> </igDP:RecordFilter.Conditions> </igDP:RecordFilter> </igDP:FieldLayout.RecordFilters> </igDP:FieldLayout> </igDP:XamDataPresenter.FieldLayouts> </igDP:XamDataPresenter>
In Visual Basic:
Imports Infragistics.Windows.DataPresenter Imports Infragistics.Windows.Controls ... Dim deptRecordFilter As New RecordFilter With {.FieldName = "department"} Dim salesDeptCondition As New ComparisonCondition(ComparisonOperator.Contains, "Sales") deptRecordFilter.Conditions.Add(salesDeptCondition) Me.xamDataPresenter1.FieldLayouts(0).RecordFilters.Add(deptRecordFilter)
In C#:
using Infragistics.Windows.DataPresenter; using Infragistics.Windows.Controls; ... RecordFilter deptRecordFilter = new RecordFilter { FieldName = "department" }; ComparisonCondition salesDeptCondition = new ComparisonCondition(ComparisonOperator.Contains, "Sales"); deptRecordFilter.Conditions.Add(salesDeptCondition); this.xamDataPresenter1.FieldLayouts[0].RecordFilters.Add(deptRecordFilter);