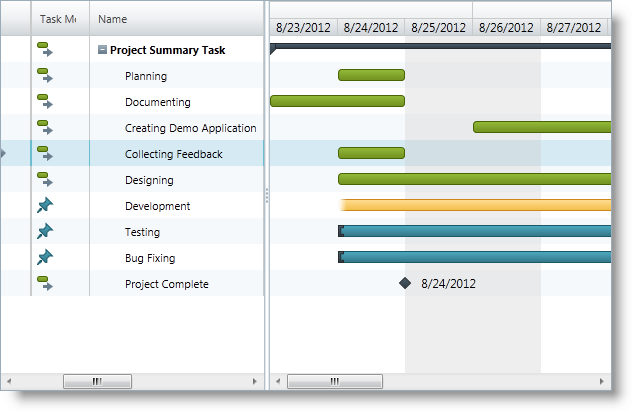
This topic describes how to add the xamGantt™ control to a page.
This topic contains the following sections:
The procedure explains how you can add the xamGantt control to a page and which required references you should include in your project.
The following screenshot previews the result.
To complete the procedure, you need the following:
A project with the following required NuGet package reference:
Infragistics.WPF.Gantt
For more information on setting up the NuGet feed and adding NuGet packages, you can take a look at the following documentation: NuGet Feeds.
A project with the ProjectDataHelper class containing sample project data.
Following is a conceptual overview of the process:
The following steps demonstrate how to add xamGantt control to a page and bind it to sample project data.
Add the required namespaces
Add the following namespaces:
In XAML:
xmlns:ig="http://schemas.infragistics.com/xaml"
xmlns:local="clr-namespace:[Your ViewModel Namespace]"
Create a ViewModel class
Create a ViewModel class that exposes a public property Project of type Project. This class inherits ObservableModel class that implements the INotifyPropertyChanged interface. The project sample data is loaded in the class constructor.
See the Code Example: ProjectViewModel class for more details.
Add xamGantt control to the page
Add the xamGantt control in the page Grid container and bind it to the project data provided by the ProjectViewModel:
In XAML:
<Grid>
<Grid.Resources>
<!--Create a static Grid resource of type ProjectViewModel-->
<local:ProjectViewModel x:Key="viewmodel" />
</Grid.Resources>
<Grid.DataContext>
<!--Bind the Grid DataContext
to the created data resource -->
<Binding Source="{StaticResource viewmodel}" />
</Grid.DataContext>
<!— Add a xamGantt and bind it to the data -->
<ig:XamGantt x:Name="gantt"
Project="{Binding Project}"/>
</Grid>
The ProjectViewModel class exposes a public property Project of type Project
. This property is initialized and populated with sample project data in the constructor of the ViewModel class.
In C#:
using System;
using System.ComponentModel;
using Infragistics.Controls.Schedules;
public class ProjectViewModel : ObservableModel
{
public ProjectViewModel()
{
this._project = ProjectDataHelper.GenerateProjectData();
}
private Project _project;
public Project Project
{
get
{
return this._project;
}
set
{
if (this._project != value)
{
this._project = value;
this.NotifyPropertyChanged("Project");
}
}
}
}
public class ObservableModel : INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
protected void NotifyPropertyChanged(String info)
{
if (PropertyChanged != null)
{
PropertyChanged(this, new PropertyChangedEventArgs(info));
}
}
}
In Visual Basic:
Imports System.ComponentModel
Imports Infragistics.Controls.Schedules
Public Class ProjectViewModel
Inherits ObservableModel
Public Sub New()
Me._project = ProjectDataHelper.GenerateProjectData()
End Sub
Private _project As Project
Public Property Project() As Project
Get
Return Me._project
End Get
Set(value As Project)
Me._project = value
Me.NotifyPropertyChanged("Project")
End Set
End Property
End Class
Public Class ObservableModel
Implements INotifyPropertyChanged
Public Event PropertyChanged(ByVal sender As Object, ByVal e As PropertyChangedEventArgs) Implements INotifyPropertyChanged.PropertyChanged
Protected Overridable Sub NotifyPropertyChanged(ByVal propertyName As String)
RaiseEvent PropertyChanged(Me, New PropertyChangedEventArgs(propertyName))
End Sub
End Class
The following topics provide additional information related to this topic.