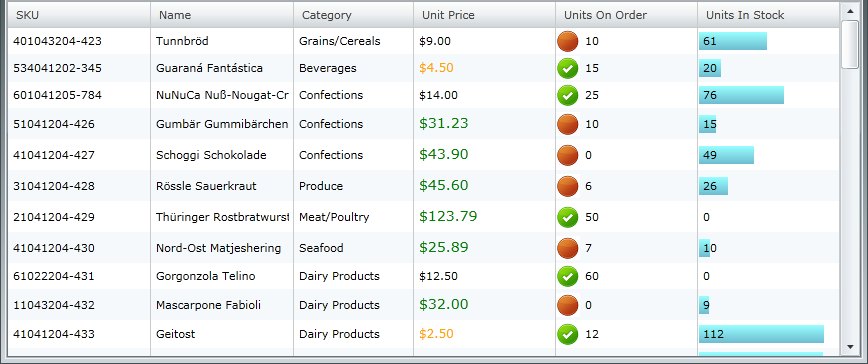
Please note that this control has been retired and is now obsolete to the XamDataGrid control, and as such, we recommend migrating to that control. It will not be receiving any new features, bug fixes, or support going forward. For help or questions on migrating your codebase to the XamDataGrid, please contact support.
Conditional formatting allows you to test certain values, such as a column of cells in xamGrid™, and apply specific appearances to any of those cells that match certain criteria. For instance, you can build a condition that will check if cell values are below a certain number (such as zero) and apply a style to those cells.
Columns can have multiple conditions applied to them, each condition being evaluated in the order defined, and conditions can be grouped into single evaluation elements.
Enable Conditional Formatting
You can enable conditional formatting by setting ConditionalFormattingSettings object’s AllowConditionalFormatting property to True.
Off of each Column is a collection which holds ConditionalFormat rules. Rules are applied to all row islands that share the Column, rules are not applied for each individual row islands.
The following code demonstrates how set up the DataBarConditionalFormatRule UnitPrice column of the xamGrid control.
In XAML:
<ig:XamGrid Grid.Row="0" x:Name="MyDataGrid" AutoGenerateColumns="False"> <ig:XamGrid.ConditionalFormattingSettings> <ig:ConditionalFormattingSettings AllowConditionalFormatting="True"/> </ig:XamGrid.ConditionalFormattingSettings> <ig:XamGrid.Columns> <ig:TextColumn Key="ProductID"/> <ig:TextColumn Key="ProductName"/> <ig:TextColumn Key="QuantityPerUnit"/> <ig:TextColumn Key="UnitPrice"/> <!-- Add Conditional Format Rule to Column --> <ig:TextColumn Key="UnitsInStock"> <ig:TextColumn.ConditionalFormatCollection> <ig:DataBarConditionalFormatRule DataBarDirection="Bidirectional" DataBrush="Red" MaximumValue="50" MinimumValue="0" StyleScope="Cell" NegativeDataBrush="Yellow" UseNegativeDataBar="True"> </ig:DataBarConditionalFormatRule> </ig:TextColumn.ConditionalFormatCollection> </ig:TextColumn> <ig:TextColumn Key="UnitsOnOrder"/> <ig:TextColumn Key="ReorderLevel"/> <ig:TextColumn Key="Discontinued"/> </ig:XamGrid.Columns> </ig:XamGrid>
In Visual Basic:
' Enable Conditional Formatting Me.MyDataGrid.ConditionalFormattingSettings.AllowConditionalFormatting = true Dim MyRule As New DataBarConditionalFormatRule MyRule.DataBarDirection = DataBarDirection.Bidirectional MyRule.DataBrush = New SolidColorBrush(Colors.Red) MyRule.NegativeDataBrush = New SolidColorBrush(Colors.Yellow) MyRule.StyleScope = StyleScope.Cell MyRule.UseNegativeDataBar = true ' Add Rule to the UnitsInStock Column Me.MyDataGrid.Columns.DataColumns("UnitsInStock").ConditionalFormatCollection.Add(MyRule)
In C#:
// Enable Conditional Formatting this.MyDataGrid.ConditionalFormattingSettings.AllowConditionalFormatting = true; // Create DataBarConditionFormatRule DataBarConditionalFormatRule MyRule = new DataBarConditionalFormatRule(); MyRule.DataBarDirection = DataBarDirection.Bidirectional; MyRule.DataBrush = new SolidColorBrush(Colors.Red); MyRule.NegativeDataBrush = new SolidColorBrush(Colors.Yellow); MyRule.StyleScope = StyleScope.Cell; MyRule.UseNegativeDataBar = true; // Add Rule to the UnitsInStock Column this.MyDataGrid.Columns.DataColumns["UnitsInStock"].ConditionalFormatCollection.Add(MyRule);
There are no default styles for each of the conditional formatting rules, you have to define the styles yourself.
There is no default icons defined for the IconConditionalFormatRule. You will need to define your own set of icons. The icons are DataTemplates.
You can have multiple rules defined in a ConditionalFormatCollection which can all change the same aspect of a style.
Precedence is given from top to bottom with the top element given the top priority. For example if you have the following two rules defined in your ConditionalFormatCollection:
In XAML:
<ig:GreaterThanConditionalFormatRule Value="5" StyleToApply="{StaticResource Style1}"/> <ig:GreaterThanConditionalFormatRule Value="10" StyleToApply="{StaticResource Style2}"/>
If the current cell value is 30, both rules will evaluate as true. However, the first rule gets priority so the cell will be set to Style1.
Setting the IsTerminalRule property on a condition means that when that condition is evaluated, the following conditions will not be evaluated.
In the following example, when the second condition is reached, the third condition is never evaluated.
In XAML:
<ig:XamGrid Grid.Row="0" x:Name="MyDataGrid" AutoGenerateColumns="False"> <ig:XamGrid.ConditionalFormattingSettings> <ig:ConditionalFormattingSettings AllowConditionalFormatting="True"/> </ig:XamGrid.ConditionalFormattingSettings> <ig:XamGrid.Columns> <ig:TextColumn Key="ProductID"/> <ig:TextColumn Key="ProductName"/> <ig:TextColumn Key="QuantityPerUnit"/> <ig:TextColumn Key="UnitPrice"/> <ig:TextColumn Key="UnitsInStock"> <ig:TextColumn.ConditionalFormatCollection> <ig:GreaterThanConditionalFormatRule Value="5" StyleToApply="{StaticResource Style1}" /> <ig:GreaterThanConditionalFormatRule Value="10" IsTerminalRule="True" StyleToApply="{StaticResource Style2}" /> <ig:GreaterThanConditionalFormatRule Value="15" StyleToApply="{StaticResource Style3}" /> </ig:TextColumn.ConditionalFormatCollection> </ig:TextColumn> <ig:TextColumn Key="UnitsOnOrder"/> <ig:TextColumn Key="ReorderLevel"/> <ig:TextColumn Key="Discontinued"/> </ig:XamGrid.Columns> </ig:XamGrid>
Related Topic