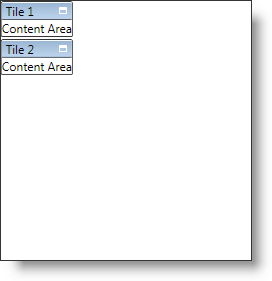
You can add xamTileManager™ to a page using a layout container and adding the control to the Children collection of the layout container.
You will add xamTileManager to your page. When you run the finished project, you should see a xamTileManager in your page that looks similar to the screen shot below.
Create a WPF project.
Add the following NuGet package to your application:
Infragistics.WPF.TileManager
For more information on setting up the NuGet feed and adding NuGet packages, you can take a look at the following documentation: NuGet Feeds.
Place using/Imports directives in your code-behind or add an XML namespace definition for xamTileManager.
In XAML:
xmlns:ig="http://schemas.infragistics.com/xaml"
In Visual Basic:
Imports Infragistics.Controls.Layouts
In C#:
using Infragistics.Controls.Layouts;
Name the default Grid layout panel in the Page so that you can reference it in the code-behind.
In XAML:
<Grid Name="LayoutRoot"> </Grid>
Add an instance of the xamTileManager to the default Grid layout panel. If you are doing this in procedural code, you can handle the Page Loaded event and place the code in the event handler.
In XAML:
<ig:XamTileManager Name="xamTileManager1"> <!--TODO: Add XamTile objects here--> </ig:XamTileManager>
In Visual Basic:
Dim xamTileManager1 As XamTileManager Private Sub Loaded(ByVal sender As Object, ByVal e As RoutedEventArgs) xamTileManager1 = New XamTileManager() Me.LayoutRoot.Children.Add(xamTileManager1) ' TODO: Add XamTile objects to xamTileManager End Sub
In C#:
private XamTileManager xamTileManager1; private void Loaded(object sender, RoutedEventArgs e) { xamTileManager1 = new XamTileManager(); this.LayoutRoot.Children.Add(xamTileManager1); // TODO: Add XamTile objects to xamTileManager }
In XAML:
<ig:XamTile Header="Tile 1" Content="Content Area" />
In Visual Basic:
Dim tile1 As New XamTile With _ {.Header = "Tile 1", .Content = "Content Area"} Me.xamTileManager1.Items.Add(tile1)
In C#:
XamTile tile1 = new XamTile { Header = "Tile 1", Content = "Content Area" }; this.xamTileManager1.Items.Add(tile1);
Add a second XamTile object to xamTileManager’s Items collection.
Set its Header property to "Tile 2".
Set its Content property to "Content Area".
In XAML:
<ig:XamTile Header="Tile 2" Content="Content Area" />
In Visual Basic:
Dim tile2 As New XamTile With _ {.Header = "Tile 2", .Content = "Content Area"} Me.xamTileManager1.Items.Add(tile2)
In C#:
XamTile tile2 = new XamTile { Header = "Tile 2", Content = "Content Area" }; this.xamTileManager1.Items.Add(tile2);
Run the project.