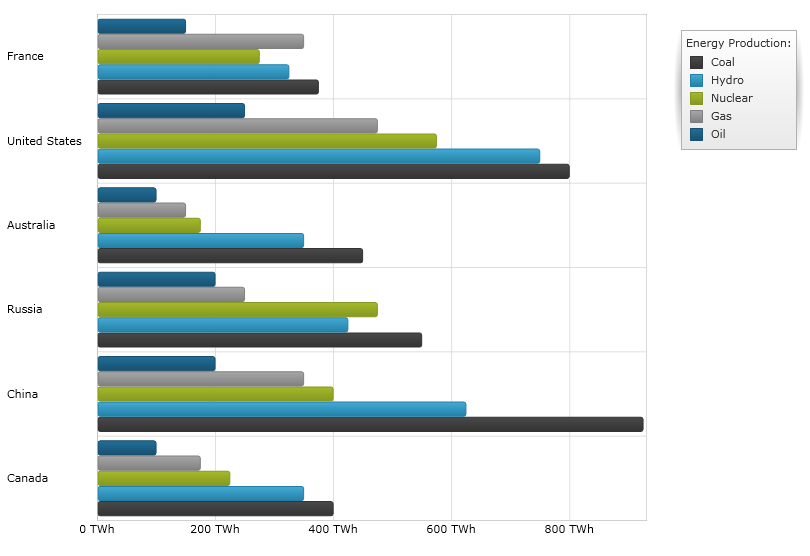
This topic explains, with code example, how to use the BarSeries in the XamDataChart™ control.
The topic is organized as follows:
Data Binding
Corners of Bar Series
Bar Series belongs to a group of Category Series and it is rendered using a collection of rectangles that extend from the left to right of the chart towards the values of data points. Bar Series uses the same concepts of data plotting as Column Series but data points are stretched along a vertical line (y-axis) rather than horizontal line (x-axis). In other words, the BarSeries is rendered like the ColumnSeries but with 90 degrees clockwise rotation. For more conceptual information and comprehension with other types of series and supported types of axes, refer to the Category Series and Chart Axes topics.
Figures 1 and 2 demonstrate how the BarSeries and the ColumnSeries look when plotted in the XamDataChart control.
Figure 1: Sample implementation of the BarSeries series type.
Figure 2: Sample implementation of the ColumnSeries series type.
Although the XamDataChart supports plotting unlimited number of various types of series, it is recommended to use the BarSeries with similar types of series. Refer to the Multiple Series topic for information on what types of series are recommended with the BarSeries and how to plot multiple Ba types of series.
While the XamDataChart control allows you to easily bind it to your own data model, it is important that you are supplying the appropriate amount and type of data that the series requires. If the data does not meet the minimum requirements based on the type of series that you are using, an error will be generated. Refer to the Series Requirements and Category Series topics for more information on data series requirements.
The following is a list of data requirements for the BarSeries
type:
The data model must contain at least one numeric property.
The data model may contain an optional string or date time property for labels.
The data source should contain at least one data item.
The BarSeries
renders data using the following rules:
Each row in the data column specified as the ValueMemberPath property of the data mapping is drawn as a separate horizontal bar.
The string or date time column that is specified as the Label property of data mapping on the y-axis is used as the category labels. If the Label token is not specified, default labels are used.
Category labels are drawn on the y-axis. Data values are drawn on the x-axis.
When rendering, multiple series of the BarSeries
type that share the same x-axis (see the Multiple Series topic) is rendered in clusters where each cluster represents a row of data. The first series in the Series
collection of the XamDataChart control renders as a bar on the bottom of the cluster. Each successive series is rendered on the top of the previous series. However, if a series set does not share the x-axis, they are rendered in layers with each successive series rendered in front of the previous one.
The BarSeries
type supports plotting their bar elements on the left or right side of specific reference value using the ReferenceValue property of the x-axis. For more information on this feature refer to the Axis Reference Value topic.
The code snippet below shows how to bind the BarSeries object to a sample of category data (which is available for download from Sample Energy Data resource). Refer to the data requirements section of this topic for information about data requirements for the BarSeries.
In XAML:
xmlns:local="clr-namespace:Infragistics.Models;assembly=YourAppName"
...
<ig:XamDataChart x:Name="DataChart" >
<ig:XamDataChart.Resources>
<ResourceDictionary>
<local:EnergyDataSource x:Key="data" />
</ResourceDictionary>
</ig:XamDataChart.Resources>
<ig:XamDataChart.Axes>
<ig:NumericXAxis x:Name="XAxis" />
<ig:CategoryYAxis x:Name="YAxis" ItemsSource="{StaticResource data}"
Label="Country" />
</ig:XamDataChart.Axes>
<ig:XamDataChart.Series>
<ig:BarSeries ItemsSource="{StaticResource data}" ValueMemberPath="Coal" Title="Coal"
XAxis="{x:Reference XAxis}"
YAxis="{x:Reference YAxis}">
</ig:BarSeries>
<ig:BarSeries ItemsSource="{StaticResource data}" ValueMemberPath="Hydro" Title="Hydro"
XAxis="{x:Reference XAxis}"
YAxis="{x:Reference YAxis}">
</ig:BarSeries>
<ig:BarSeries ItemsSource="{StaticResource data}" ValueMemberPath="Nuclear" Title="Nuclear"
XAxis="{x:Reference XAxis}"
YAxis="{x:Reference YAxis}">
</ig:BarSeries>
<ig:BarSeries ItemsSource="{StaticResource data}" ValueMemberPath="Gas" Title="Gas"
XAxis="{x:Reference XAxis}"
YAxis="{x:Reference YAxis}">
</ig:BarSeries>
<ig:BarSeries ItemsSource="{StaticResource data}" ValueMemberPath="Oil" Title="Oil"
XAxis="{x:Reference XAxis}"
YAxis="{x:Reference YAxis}">
</ig:BarSeries>
</ig:XamDataChart.Series>
</ig:XamDataChart>
In C#:
var data = new EnergyDataSource();
var xAxis = new NumericXAxis();
var yAxis = new CategoryYAxis();
yAxis.ItemsSource = data;
yAxis.Label = "Country";
var series = new BarSeries();
series.ItemsSource = data;
series.ValueMemberPath = "Coal";
series.Title = "Coal";
series.XAxis = xAxis;
series.YAxis = yAxis;
var chart = new XamDataChart();
chart.Axes.Add(xAxis);
chart.Axes.Add(yAxis);
chart.Series.Add(series);
Figure 3: The BarSeries series type with rectangular corners.
Bar Series allow setting corners of visual bar elements using the RadisuX and RadiusY properties. Increasing values of these properties will curve the corners of bar elements while decreasing the value makes bars more rectangular. The following code snippet demonstrates how to set round corners on the BarSeries.
In XAML:
<ig:BarSeries RadiusX="10" RadiusY="10" />
In C#:
var series = new BarSeries();
series.RadiusX = 10;
series.RadiusY = 10;
...
DataChart.Series.Add(series);
Figure 4: The BarSeries series type with round corners.