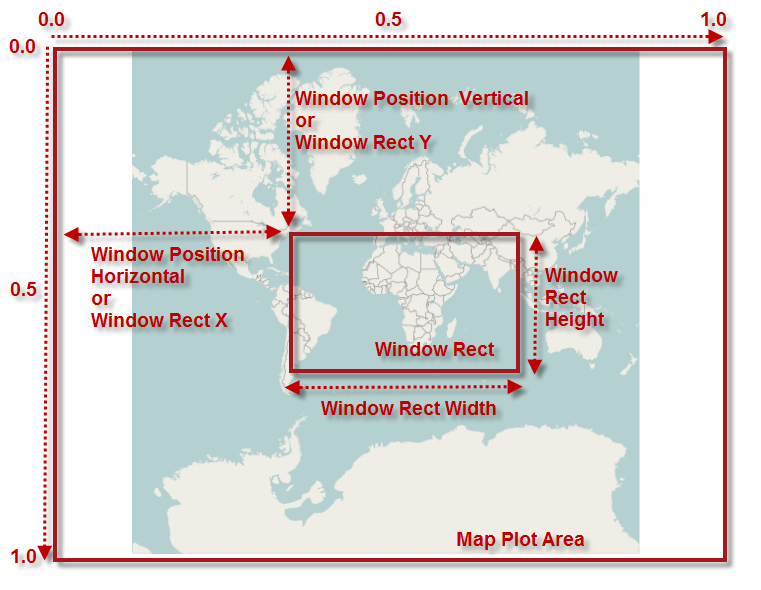
This topic provides information about navigating the map content in the UltraGeographicMap™ control using code.
The following table lists the topics required as a prerequisite to understanding this topic.
This topic contains the following sections:
In the UltraGeographicMap control, there are two navigation techniques for navigating the map content using code. The first technique allows map navigation using window navigation system and the second one using world navigation system.
Window Navigation System
World Navigation System
The UltraGeographicMap control provides methods for converting values between both navigation systems. These methods are explained in the Examples section of this topic.
The window navigation system consists of properties for setting position and size of WindowRect – a map view in window coordinates. In this navigation system values can be set between 0 and 1 for properties of the map window view.
The following image is a preview of UltraGeographicMap control with highlighted position and size of the WindowRect when zoomed to some region of the map content (for example, Africa and Europe continents).
The following table summarizes properties for setting position and size of map window in the UltraGeographicMap control.
The world navigation system consists of properties for setting position and size of the WorldRect – a map view in geographic coordinates. In this navigation system values can be set between -180 and 180 for longitude properties of the WorldRect and values between -85 and 85 for latitude properties of the WorldRect view.
The following image is a preview of UltraGeographicMap control with highlighted position and size of the WorldRect when zoomed to some region of the map content (for example, Africa and Europe continents).
The following table summarizes properties for setting position and size of map window in the UltraGeographicMap control.
In C#:
var widthScale = (0.1 * this.GeoMap.WindowRect.Width);
var heightScale = (0.1 * this.GeoMap.WindowRect.Height);
var x = this.GeoMap.WindowRect.X + (widthScale / 2);
var y = this.GeoMap.WindowRect.Y + (heightScale / 2);
var w = this.GeoMap.WindowRect.Width - widthScale;
var h = this.GeoMap.WindowRect.Height - heightScale;
this.GeoMap.WindowRect = new Rectangle(x, y, w, h);
In Visual Basic:
Dim widthScale = (0.1 * Me.GeoMap.WindowRect.Width)
Dim heightScale = (0.1 * Me.GeoMap.WindowRect.Height)
Dim x = Me.GeoMap.WindowRect.X + (widthScale / 2)
Dim y = Me.GeoMap.WindowRect.Y + (heightScale / 2)
Dim w = Me.GeoMap.WindowRect.Width - widthScale
Dim h = Me.GeoMap.WindowRect.Height - heightScale
Me.GeoMap.WindowRect = New Rectangle(x, y, w, h)
In C#:
var widthScale = (0.1 * this.GeoMap.WindowRect.Width);
var heightScale = (0.1 * this.GeoMap.WindowRect.Height);
var x = this.GeoMap.WindowRect.X - (widthScale / 2);
var y = this.GeoMap.WindowRect.Y - (heightScale / 2);
var w = this.GeoMap.WindowRect.Width + widthScale;
var h = this.GeoMap.WindowRect.Height + heightScale;
this.GeoMap.WindowRect = new Rectangle(x, y, w, h);
In Visual Basic:
Dim widthScale = (0.1 * Me.GeoMap.WindowRect.Width)
Dim heightScale = (0.1 * Me.GeoMap.WindowRect.Height)
Dim x = Me.GeoMap.WindowRect.X - (widthScale / 2)
Dim y = Me.GeoMap.WindowRect.Y - (heightScale / 2)
Dim w = Me.GeoMap.WindowRect.Width + widthScale
Dim h = Me.GeoMap.WindowRect.Height + heightScale
Me.GeoMap.WindowRect = New Rectangle(x, y, w, h)
In C#:
this.GeoMap.WindowRect = new Rectangle(0.2, 0.3, 0.6, 0.4);
In Visual Basic:
Me.GeoMap.WindowRect = New Rectangle(0.2, 0.3, 0.6, 0.4)
In C#:
var geoRegion = new Rectangle(-30, -40, 120, 80);
this.GeoMap.WindowRect = this.GeoMap.GetZoomFromGeographic(geoRegion);
In Visual Basic:
Dim geoRegion = New Rectangle(-30, -40, 120, 80)
Me.GeoMap.WindowRect = Me.GeoMap.GetZoomFromGeographic(geoRegion)
In C#:
this.GeoMap.WindowRect = Rectangle(0.0, 0.0, 1.0, 1.0);
In Visual Basic:
Me.GeoMap.WindowRect = New Rectangle(0.0, 0.0, 1.0, 1.0)
In C#:
var geoRegion = new Rectangle(-180, -75, 360, 150);
this.GeoMap.WindowRect = this.GeoMap.GetZoomFromGeographic(geoRegion);
In Visual Basic:
Dim geoRegion = New Rectangle(-180, -75, 360, 150)
Me.GeoMap.WindowRect = Me.GeoMap.GetZoomFromGeographic(geoRegion)
In C#:
this.GeoMap.WorldRect = new Rectangle(-30, -40, 120, 80);
In Visual Basic:
Me.GeoMap.WorldRect = New Rectangle(-30, -40, 120, 80)
In C#:
this.GeoMap.WindowPositionHorizontal = this.GeoMap.WindowRect.X - 0.05;
In Visual Basic:
Me.GeoMap.WindowPositionHorizontal = Me.GeoMap.WindowRect.X - 0.05
In C#:
this.GeoMap.WindowPositionHorizontal = this.GeoMap.WindowRect.X + 0.05;
In Visual Basic:
Me.GeoMap.WindowPositionHorizontal = Me.GeoMap.WindowRect.X + 0.05
In C#:
this.GeoMap.WindowPositionVertical = this.GeoMap.WindowRect.Y - 0.05;
In Visual Basic:
Me.GeoMap.WindowPositionVertical = Me.GeoMap.WindowRect.Y - 0.05
In C#:
this.GeoMap.WindowPositionVertical = this.GeoMap.WindowRect.Y + 0.05;
In Visual Basic:
Me.GeoMap.WindowPositionVertical = Me.GeoMap.WindowRect.Y + 0.05
The following topics provide additional information related to this topic.