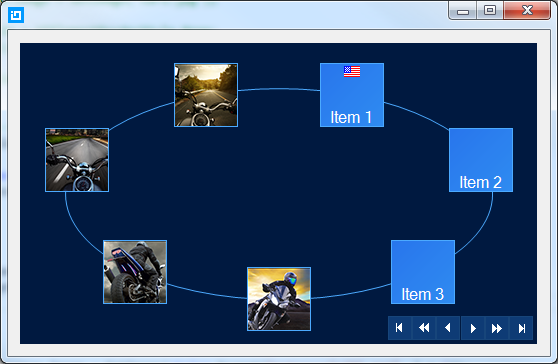
This topic demonstrates how to add UltraCarousel items using the Code-Behind.
This topic contains the following sections:
In this topic, you will learn how to add the UltraCarousel control and configure it through the control’s properties in code. The control has a DataSource property that can be used to bind any collection of strings or images that would display on the items.
Install the Infragistics WinForms 2014 Volume 2 or later version of the product.
Start with a new Windows Forms application using Visual Studio 2010 or later version.
The following code example demonstrates creating an instance of UltraCarousel control and adding it to the form. By default the control will display with an item path and scroll buttons before adding any items, or binding to a data.
In C#:
UltraCarousel carousel1 = new UltraCarousel();
this.Controls.Add(carousel1);
In Visual Basic:
Dim carousel1 As New UltraCarousel()
Me.Controls.Add(carousel1)
An Item in the UltraCarousel control is the visual representations of the data object. Items appear as square tiles on the path. The ItemSlots property determines the number of items ( Default=5 ) displayed on the path. The default size of each item is 64x64 (In pixels).
The following code example demonstrates manually adding carousel items, and setting the item appearance with images.
In C#:
for (int i = 0; i < 5; i++)
{
CarouselItem item = new CarouselItem();
item.Settings.Appearance.Image = GetImage(string.Format("motorcycle{0}.png", i + 1));
carousel1.Items.Add(item);
}
In Visual Basic:
For i As Integer = 0 To 4
Dim item As New CarouselItem()
item.Settings.Appearance.Image = GetImage(String.Format("motorcycle{0}.png", i + 1))
carousel1.Items.Add(item)
Next
In addition to adding items manually, the UltraCarousel control supports data binding.
This code demonstrates binding the control to a data using its DataSource property.
In C#:
carousel1.DataSource = new TestData();
In Visual Basic:
carousel1.DataSource = New TestData()
This is the test data used in the data binding. With this test data model you may need to add some images in your project and set their Build Action
to Embedded Resources .
In C#:
public class TestData : List<TestDataItem>
{
public TestData()
{
for (int i = 0; i < 5; i++)
{
Add(new TestDataItem { Item = "Item", img = Images.GetImage(string.Format("motorcycle{0}.png", i + 1)) });
}
}
}
public class TestDataItem
{
public string Item { get; set; }
public Image img { get; set; }
}
public class Images
{
public static Image GetImage(string imageName)
{
var type = typeof(Form1);
var resName = string.Format("{0}", imageName);
var stream = type.Module.Assembly.GetManifestResourceStream(type, resName);
return null == stream ? null : Image.FromStream(stream);
}
}
In Visual Basic:
Public Class TestData
Inherits List(Of TestDataItem)
Public Sub New()
For i As Integer = 0 To 4
Add(New TestDataItem() With { _
.Item = "Item", _
.img = Images.GetImage(String.Format("motorcycle{0}.png", i + 1)) _
})
Next
End Sub
End Class
Public Class TestDataItem
Public Property Item() As String
Get
Return m_Item
End Get
Set
m_Item = Value
End Set
End Property
Private m_Item As String
Public Property img() As Image
Get
Return m_img
End Get
Set
m_img = Value
End Set
End Property
Private m_img As Image
End Class
Public Class Images
Public Shared Function GetImage(imageName As String) As Image
Dim type = GetType(Form1)
Dim resName = String.Format("{0}", imageName)
Dim stream = type.[Module].Assembly.GetManifestResourceStream(type, resName)
Return If(stream Is Nothing, Nothing, Image.FromStream(stream))
End Function
End Class
The following topics provide additional information related to this topic.