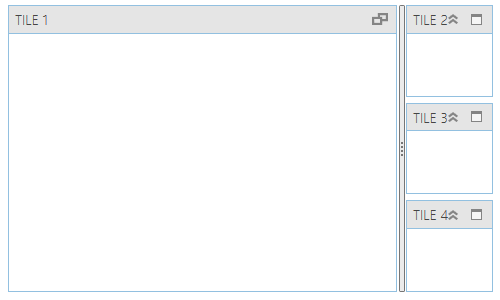
This topic describes how to extend an existing control theme using Infragistics ThemeManager™.
The following topics are prerequisites to understanding this topic:
This topic contains the following sections:
The following procedure walks you through the extending of the Office2013 theme of xamTileManager using ThemeManager.
The following screenshot is a preview of the final result.
Following are the general requirements for extending the Office2013 theme of XamTileManager.
NuGet package references:
Infragistics.WPF.TileManager
Infragistics.WPF.Themes.Office2013
For more information on setting up the NuGet feed and adding NuGet packages, you can take a look at the following documentation: NuGet Feeds.
Namespaces:
A reference to the Infragistics® namespace: (xmlns:ig="http://schemas.infragistics.com/xaml")
To complete the procedure, you need the following:
A Microsoft® Visual Studio® WPF project with a page
The required NuGet package references and namespaces added to the project (See the Requirements section above.)
Include the following theme resources in your project:
Following is a conceptual overview of the process:
The following steps demonstrate how to extend and apply the modified Office2013 theme to the xamTileManager control.
Create a theme for xamTileManager in Resource Dictionary
Refer the ExtendedTheme.xamTileManager.xaml for more information.
Create an extended theme class that derives from the Office2013Theme class
Create a class named ExtendedOffice2013Theme that derives from the Office2013Theme class:
In C#:
public class ExtendedOffice2013Theme : Office2013Theme
{
// Class implementation here
}
In Visual Basic:
Public Class ExtendedOffice2013Theme Inherits Office2013Theme
' Class implementation here
End Class
Override the ConfigureControlMappings method in your extended theme class
Override the ConfigureControlMappings method in your extended theme class:
In C#:
protected override void ConfigureControlMappings()
{
// Pre-set the mappings for all controls via a call
// to the base implementation of the ConfigureControlMappings method
base.ConfigureControlMappings();
string assemblyFullName = typeof(ExtendedOffice2013Theme).Assembly.FullName;
// Override the mapping of the XamTileManager control
Mappings[ControlMappingKeys.XamTileManager] =
ThemeBase.BuildLocationString(assemblyFullName, @"/ExtendedTheme.xamTileManager.xaml");
}
In Visual Basic:
Protected Overrides Sub ConfigureControlMappings()
' Pre-set the mappings for all controls via a call
' to the base implementation of the ConfigureControlMappings method
MyBase.ConfigureControlMappings()
Dim assemblyFullName As String = GetType(ExtendedOffice2013Theme).Assembly.FullName
' Override the mapping of the XamTileManager control
Mappings(ControlMappingKeys.XamTileManager) = _
ThemeBase.BuildLocationString(assemblyFullName, "/ExtendedTheme.xamTileManager.xaml")
End Sub
Apply your extended theme to the control
You can apply your extended theme to the control as demonstrated in the code snippet below:
In XAML:
<Grid>
<ig:ThemeManager.Theme>
<extendedTheme:ExtendedOffice2013Theme />
</ig:ThemeManager.Theme>
<ig:XamTileManager>
<ig:XamTile Header="TILE 1"
IsMaximized="True" />
<ig:XamTile Header="TILE 2" />
<ig:XamTile Header="TILE 3" />
<ig:XamTile Header="TILE 4" />
</ig:XamTileManager>
</Grid>
Following is the full code for this procedure.
Window XAML code
In XAML:
<Grid>
<ig:ThemeManager.Theme>
<extendedTheme:ExtendedOffice2013Theme />
</ig:ThemeManager.Theme>
<ig:XamTileManager>
<ig:XamTile Header="TILE 1"
IsMaximized="True" />
<ig:XamTile Header="TILE 2" />
<ig:XamTile Header="TILE 3" />
<ig:XamTile Header="TILE 4" />
</ig:XamTileManager>
</Grid>
ExtendedOffice2013Theme.cs
In C#:
public class ExtendedOffice2013Theme : Office2013Theme
{
protected override void ConfigureControlMappings()
{
// Pre-set the mappings for all controls via a call
// to the base implementation of the ConfigureControlMappings method
base.ConfigureControlMappings();
string assemblyFullName = typeof(ExtendedOffice2013Theme).Assembly.FullName;
// Override the mapping of the XamTileManager control
Mappings[ControlMappingKeys.XamTileManager] =
ThemeBase.BuildLocationString(assemblyFullName, @"/ExtendedTheme.xamTileManager.xaml");
}
}
In Visual Basic:
Public Class ExtendedOffice2013Theme Inherits Office2013Theme
Protected Overrides Sub ConfigureControlMappings()
' Pre-set the mappings for all controls via a call
' to the base implementation of the ConfigureControlMappings method
MyBase.ConfigureControlMappings()
Dim assemblyFullName As String = GetType(ExtendedOffice2013Theme).Assembly.FullName
' Override the mapping of the XamTileManager control
Mappings(ControlMappingKeys.XamTileManager) = _
ThemeBase.BuildLocationString(assemblyFullName, "/ExtendedTheme.xamTileManager.xaml")
End Sub
End Class
The following topics provide additional information related to this topic.