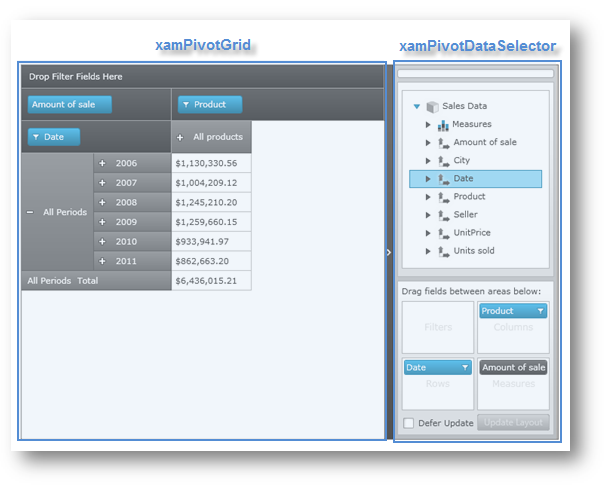
This topic demonstrates how to get started with the xamPivotGrid™ control by providing step-by-step procedure for adding this control to a WPF application. Code examples include listings in XAML as well as procedural code.
The topic is organized as follows:
Using XAML Code
Using Procedural Code
The procedures below demonstrate how to add the xamPivotGrid control with xamPivotDataSelector™ to an application, and then bind these controls to FlatDataSource with a data model. For more information on binding data and using different data source for the xamPivotGrid control refer to the Binding Data to the xamPivotGrid topic.
Following is a preview of the final result:
Figure 1: A sample of the xamPivotGrid control with xamPivotDataSelector.
Download the SalesDataSample class from the Sales Data Sample resource and add it to your application. This class is responsible to act as an item source for the FlatDataSource of the xamPivotGrid control however you can create your own data model by implementing the IEnumerable interface (e.g. List
, Collection
, Queue
, Stack
etc.). Refer to the Binding Data topic in order to provide different data source for the xamPivotGrid control.
Add the following NuGet package references to your application:
Infragistics.WPF.Controls.Grids.XamPivotGrid
Infragistics.WPF.Olap.FlatData
For more information on setting up the NuGet feed and adding NuGet packages, you can take a look at the following documentation: NuGet Feeds.
Add required namespace declarations:
In XAML:
xmlns:olap="http://schemas.infragistics.com/olap" xmlns:ig="http://schemas.infragistics.com/xaml" xmlns:models="clr-namespace:Infragistics.Samples.Data.Models"
In Visual Basic:
Imports Infragistics.Olap ' common OLAP objects Imports Infragistics.Olap.FlatData ' FlatDataSource Imports Infragistics.Controls.Grids ' xamPivotGrid Imports Infragistics.Samples.Data.Models ' SalesDataSample
In C#:
using Infragistics.Olap; // common OLAP objects using Infragistics.Olap.FlatData; // FlatDataSource using Infragistics.Controls.Grids; // xamPivotGrid using Infragistics.Samples.Data.Models; // SalesDataSample
This section provides step-by-step instructions for adding the xamPivotGrid control to your application and binding it to the SalesDataSample data through FlatDataSource view model.
Add the SalesDataSample data as a resource.
In XAML:
xmlns:models="clr-namespace:Infragistics.Samples.Data.Models" ... <models:SalesDataSample x:Key="dataSample"/>
Add the FlatDataSource view model as a resource and bind it to the SalesDataSample data.
In XAML:
<olap:FlatDataSource x:Key="dataSource" ItemsSource="{StaticResource dataSample}" />
Add the xamPivotGrid control to the layout root and bind it to the FlatDataSource view model.
In XAML:
<Grid x:Name="LayoutRoot" > <Grid.ColumnDefinitions> <ColumnDefinition Width="*" /> <ColumnDefinition Width="Auto" /> </Grid.ColumnDefinitions> <ig:XamPivotGrid x:Name="pivotGrid" DataSource="{StaticResource dataSource}" /> <!-- TODO Add xamPivotDataSelector control --> </Grid>
Add the xamPivotDataSelector control to the layout root and bind it to the FlatDataSource view model.
In XAML:
<ig:Expander Grid.Column="1"> <ig:XamPivotDataSelector x:Name="pivotDataSelector" DataSource="{StaticResource dataSource}" /> </ig:Expander>
Create an instance of the SalesDataSample class.
In Visual Basic:
Imports Infragistics.Samples.Data.Models ' SalesDataSamples ... Dim dataSample As New SalesDataSamples()
In C#:
using Infragistics.Samples.Data.Models; // SalesDataSample ... SalesDataSamples dataSample = new SalesDataSamples();
Create an instance of the FlatDataSource view model and bind it to the SalesDataSample data.
In Visual Basic:
Dim dataSource As New FlatDataSource() dataSource.ItemsSource = dataSample
In C#:
FlatDataSource dataSource = new FlatDataSource(); dataSource.ItemsSource = dataSample;
Add the xamPivotGrid control to the layout root and bind it to the FlatDataSource view model.
In Visual Basic:
Dim pivotGrid As New XamPivotGrid() pivotGrid.DataSource = dataSource Me.LayoutRoot.Children.Add(pivotGrid)
In C#:
XamPivotGrid pivotGrid = new XamPivotGrid(); pivotGrid.DataSource = dataSource; this.LayoutRoot.Children.Add(pivotGrid);
Add the xamPivotDataSelector control to the layout root and bind it to the FlatDataSource view model.
In Visual Basic:
Dim pivotDataSelector As New XamPivotDataSelector () pivotDataSelector.DataSource = dataSource Me.LayoutRoot.Children.Add(pivotDataSelector)
In C#:
XamPivotGrid pivotDataSelector = new XamPivotDataSelector (); pivotDataSelector.DataSource = dataSource; this.LayoutRoot.Children.Add(pivotDataSelector);