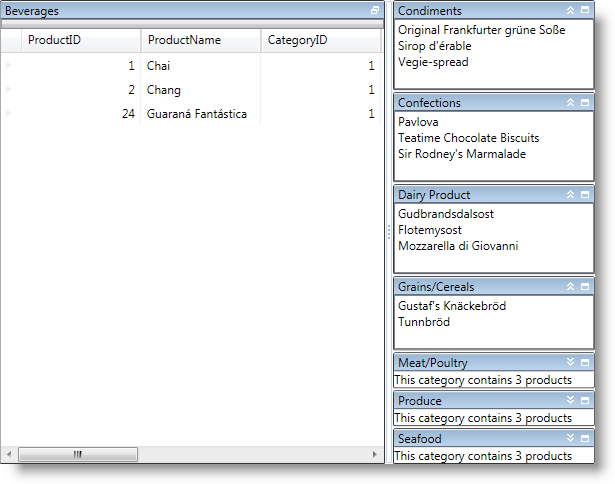
You can add tiles to xamTileManager™ by binding to a data source or by adding objects directly to the Items collection. However, just like any XAML data-bound control, you must choose between binding xamTileManager to a data source and adding items directly to the Items collection; you cannot do both .
When you bind xamTileManager to data, it will create a XamTile object for each root-level data item in your data source. However, you must create data templates that define the visual tree of the XamTile object.
You will bind xamTileManager to a collection of category data items to create a tile for each category. Each tile will display a category’s name in its header. The content in each tile will be different based on the tile’s state. In normal mode, a tile will display the category’s description. In maximized mode, a tile will display a list of products using the xamGrid™ control. In minimized mode, a tile will display the number of products contained in a category. Finally, in minimized-expanded mode, a tile will display a list of product names that belong to the category.
The DataUtil class is provided for you in C# and VB.NET for you to copy and use in your project while working through this topic.
When you run the project, you should see a xamTileManager that looks similar to the screen shot below.
Add a copy of the DataUtil class to your project.
Add tags for the page’s resource dictionary.
In XAML:
<Page.Resources> <!-- TODO: Add data templates here--> </Page.Resources>
Add a DataTemplate object to the resource dictionary. You will use this template for tiles that are in the normal state.
Set its Key property to normalTemplate.
Add a TextBlock control to the data template and bind its Text property to the Category object’s Description property.
In XAML:
<DataTemplate x:Key="normalTemplate"> <TextBlock Text="{Binding Description}" /> </DataTemplate>
Add a second DataTemplate object to the resource dictionary. You will use this template for tiles that are in the maximized state.
Set its Key property to maximizedTemplate.
Add a xamGrid control to the data template and bind its ItemsSource property to the Category object’s Products collection. If you information on the assemblies and namespaces required for using xamGrid, read Adding xamGrid to Your Page.
In XAML:
<DataTemplate x:Key="maximizedTemplate"> <ig:XamGrid ItemsSource="{Binding Products}" /> </DataTemplate>
Add a third DataTemplate object to the resource dictionary. You will use this template for tiles that are in the minimized state.
Set its Key property to minimizedTemplate.
Add a TextBlock control to the data template and bind its Text property to the Count property of the Products collection.
In XAML:
<DataTemplate x:Key="minimizedTemplate"> <TextBlock Text="{Binding Products.Count, StringFormat=This category contains {0} products}" /> </DataTemplate>
Add a fourth DataTemplate object to the resource dictionary. You will use this template for tiles that are in the minimized-expanded state.
Set its Key property to minimizedExpandedTemplate.
Add a ListBox control to the data template.
Set its ItemsSource property to the Products collection.
Set its DisplayMemberPath property to ProductName.
In XAML:
<DataTemplate x:Key="minimizedExpandedTemplate"> <ListBox ItemsSource="{Binding Products}" DisplayMemberPath="ProductName" /> </DataTemplate>
Add a xamTileManager to the default Grid panel.
Set its ItemsSource property to the CategoriesAndProducts property exposed by the DataUtil class.
Set its HeaderPath property to CategoryName.
Set its ItemTemplate property to the normal template defined in step 3.
Set its ItemTemplateMaximized property to the maximized template defined in step 4.
Set its ItemTemplateMinimized property to the minimized template defined in step 5.
Set its ItemTemplateMinimizedExpanded property to the minimized-expanded template defined in step 6.
In XAML:
<ig:XamTileManager xmlns:data="clr-namespace:IGDocumentation" Name="xamTileManager1" ItemsSource="{Binding Source={x:Static data:DataUtil.CategoriesAndProducts}}" HeaderPath="CategoryName" ItemTemplate="{StaticResource normalTemplate}" ItemTemplateMaximized="{StaticResource maximizedTemplate}" ItemTemplateMinimized="{StaticResource minimizedTemplate}" ItemTemplateMinimizedExpanded="{StaticResource minimizedExpandedTemplate}"> </ig:XamTileManager>
In Visual Basic:
Me.xamTileManager1.ItemsSource = DataUtil.CategoriesAndProducts; Me.xamTileManager1.HeaderPath = "CategoryName" Me.xamTileManager1.ItemTemplate = _ TryCast(Me.Resources("normalTemplate"), DataTemplate) Me.xamTileManager1.ItemTemplateMaximized = _ TryCast(Me.Resources("maximizedTemplate"), DataTemplate) Me.xamTileManager1.ItemTemplateMinimized = _ TryCast(Me.Resources("minimizedTemplate"), DataTemplate) Me.xamTileManager1.ItemTemplateMinimizedExpanded = _ TryCast(Me.Resources("minimizedExpandedTemplate"), DataTemplate)
In C#:
this.xamTileManager1.ItemsSource = IGDocumentation.DataUtil.CategoriesAndProducts; this.xamTileManager1.HeaderPath= "CategoryName"; this.xamTileManager1.ItemTemplate = this.Resources["normalTemplate"] as DataTemplate; this.xamTileManager1.ItemTemplateMaximized = this.Resources["maximizedTemplate"] as DataTemplate; this.xamTileManager1.ItemTemplateMinimized = this.Resources["minimizedTemplate"] as DataTemplate; this.xamTileManager1.ItemTemplateMinimizedExpanded = this.Resources["minimizedExpandedTemplate"] as DataTemplate;
Run the project.