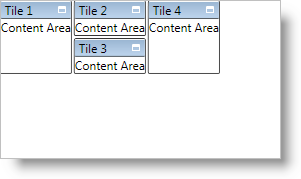
When you set the NormalModeSettings object’s TileLayoutOrder property to UseExplicitRowColumnOnTile, you are responsible for arranging XamTile objects into columns and rows by setting the XamTileManager’s attached properties - Column, ColumnSpan, Row and RowSpan. The Column, ColumnSpan, Row and RowSpan properties work just like their Grid panel counterparts. If you do not set a value for these properties, they will default to 0 just like the Grid panel. This will cause xamTileManager to stack all tiles on top of each other in the upper left-hand corner.
The following example code demonstrates how to explicitly arrange tiles.
In XAML:
<ig:XamTileManager Name="xamTileManager1"> <!--Enable explicit layout--> <ig:XamTileManager.NormalModeSettings> <ig:NormalModeSettings TileLayoutOrder="UseExplicitRowColumnOnTile" /> </ig:XamTileManager.NormalModeSettings> <ig:XamTile Header="Tile 1" Content="Content Area" ig:XamTileManager.Column="0" ig:XamTileManager.Row="0" ig:XamTileManager.RowSpan="4" ig:XamTileManager.ColumnSpan="1" /> <ig:XamTile Header="Tile 2" Content="Content Area" ig:XamTileManager.Column="1" ig:XamTileManager.Row="0" ig:XamTileManager.RowSpan="2" ig:XamTileManager.ColumnSpan="2" /> <ig:XamTile Header="Tile 3" Content="Content Area" ig:XamTileManager.Column="1" ig:XamTileManager.Row="2" ig:XamTileManager.RowSpan="2" ig:XamTileManager.ColumnSpan="2" /> <ig:XamTile Header="Tile 4" Content="Content Area" ig:XamTileManager.Column="3" ig:XamTileManager.Row="0" ig:XamTileManager.RowSpan="4" ig:XamTileManager.ColumnSpan="1" /> </ig:XamTileManager>
In Visual Basic:
Imports Infragistics.Controls.Layouts ... Me.xamTileManager1.NormalModeSettings.TileLayoutOrder = _ TileLayoutOrder.UseExplicitRowColumnOnTile Dim tile1 As New XamTile With _ {.Header = "Tile 1", .Content = "Content Area"} XamTileManager.SetColumn(tile1, 0) XamTileManager.SetRow(tile1, 0) XamTileManager.SetRowSpan(tile1, 4) XamTileManager.SetColumnSpan(tile1, 4) Dim tile2 As New XamTile With _ {.Header = "Tile 2", .Content = "Content Area"} XamTileManager.SetColumn(tile1, 1) XamTileManager.SetRow(tile1, 0) XamTileManager.SetRowSpan(tile1, 2) XamTileManager.SetColumnSpan(tile1, 2) Dim tile3 As New XamTile With _ {.Header = "Tile 3", .Content = "Content Area"} XamTileManager.SetColumn(tile1, 1) XamTileManager.SetRow(tile1, 2) XamTileManager.SetRowSpan(tile1, 2) XamTileManager.SetColumnSpan(tile1, 2) Dim tile4 As New XamTile With _ {.Header = "Tile 4", .Content = "Content Area"} XamTileManager.SetColumn(tile1, 3) XamTileManager.SetRow(tile1, 0) XamTileManager.SetRowSpan(tile1, 4) XamTileManager.SetColumnSpan(tile1, 1) Me.xamTileManager1.Items.Add(tile1) Me.xamTileManager1.Items.Add(tile2) Me.xamTileManager1.Items.Add(tile3) Me.xamTileManager1.Items.Add(tile4) ...
In C#:
using Infragistics.Controls.Layouts; ... this.xamTileManager1.NormalModeSettings.TileLayoutOrder = TileLayoutOrder.UseExplicitRowColumnOnTile; XamTile tile1 = new XamTile { Header = "Tile 1", Content = "Content Area" }; XamTileManager.SetColumn(tile1, 0); XamTileManager.SetRow(tile1, 0); XamTileManager.SetRowSpan(tile1, 4); XamTileManager.SetColumnSpan(tile1, 4); XamTile tile2 = new XamTile { Header = "Tile 2", Content = "Content Area" }; XamTileManager.SetColumn(tile1, 1); XamTileManager.SetRow(tile1, 0); XamTileManager.SetRowSpan(tile1, 2); XamTileManager.SetColumnSpan(tile1, 2); XamTile tile3 = new XamTile { Header = "Tile 3", Content = "Content Area" }; XamTileManager.SetColumn(tile1, 1); XamTileManager.SetRow(tile1, 2); XamTileManager.SetRowSpan(tile1, 2); XamTileManager.SetColumnSpan(tile1, 2); XamTile tile4 = new XamTile { Header = "Tile 4", Content = "Content Area" }; XamTileManager.SetColumn(tile1, 3); XamTileManager.SetRow(tile1, 0); XamTileManager.SetRowSpan(tile1, 4); XamTileManager.SetColumnSpan(tile1, 1); this.xamTileManager1.Items.Add(tile1); this.xamTileManager1.Items.Add(tile2); this.xamTileManager1.Items.Add(tile3); this.xamTileManager1.Items.Add(tile4); ...