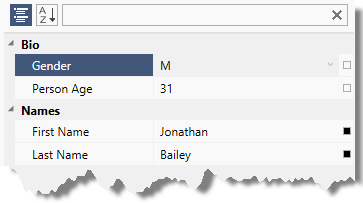
This topic explains how to define default property value and how to customize the options menu.
The following topics are prerequisites to understanding this topic:
This topic contains the following sections:
The property value reset feature of the xamPropertyGrid control allows the user to reset each property independently to its default value if a default value has been defined. Each property has a small glyph on the right side of the property value. The following screenshot shows the states of the reset value glyph:
After clicking the reset glyph an options menu will appear. By default it contains only one option for resetting property value, however you can specify a custom options menu by setting the xamPropertyGrid’s OptionsMenu property to any ContextMenu
. The DataContext
of the custom ContextMenu
will be automatically set to the PropertyGridPropertyItem associated with the current property.
The following screenshot shows the default options menu shown after clicking on the "full square" reset property value glyph:
The following table explains briefly the ways to define default property value. Further details are available after the table.
You can define a default property value using in code using the "DefaultValue" attribute.
The following code snippet demonstrates how to define a property default value using the "DefaultValue" attribute.
In C#:
public class Person
{
[DefaultValue("first-name-default-value")]
public string FirstName { get; set; }
…
}
In Visual Basic:
Public Class Person
<DefaultValue("first-name-default-value")> _
Public Property FirstName() As String
Get
Return m_FirstName
End Get
Set
m_FirstName = Value
End Set
End Property
Private m_FirstName As String
End Class
If a property has a default value that cannot be expressed with a "DefaultValue" attribute, you can provide a default property value in code using the "Reset/ShouldSerialize" pattern:
Define a "reset" method for each property you want to reset with the same name as the property prefixed by "Reset" and a return type of void. In this method set the property default value to its default.
Define a "should serialize" method for each property you want to reset with the same name as the property prefixed by "ShouldSerialize" and a return type of bool
. This method should return true
when the property value can be reset.
The following code snippet demonstrates how to define a property default value using methods.
In C#:
public class Person : INotifyPropertyChanged
{
private string firstNameDefault = "first-name-default-value";
public string FirstName { get; set; }
public void ResetFirstName()
{
this.FirstName = this.firstNameDefault;
this.PropertyChange("FirstName");
}
public bool ShouldSerializeFirstName()
{
return !this.firstNameDefault.Equals(this.FirstName);
}
…
public event PropertyChangedEventHandler PropertyChanged;
private void PropertyChange(string propertyName)
{
if (this.PropertyChanged != null)
{
this.PropertyChanged(this, new PropertyChangedEventArgs(propertyName));
}
}
}
In Visual Basic:
Public Class Person Implements INotifyPropertyChanged
Private firstNameDefault As String = "first-name-default-value"
Public Property FirstName() As String
Get
Return m_FirstName
End Get
Set
m_FirstName = Value
End Set
End Property
Private m_FirstName As String
Public Sub ResetFirstName()
Me.FirstName = Me.firstNameDefault
Me.PropertyChange("FirstName")
End Sub
Public Function ShouldSerializeFirstName() As Boolean
Return Not Me.firstNameDefault.Equals(Me.FirstName)
End Function
…
Public Event PropertyChanged As PropertyChangedEventHandler
Private Sub PropertyChange(propertyName As String)
RaiseEvent PropertyChanged(Me, New PropertyChangedEventArgs(propertyName))
End Sub
End Class
The default options menu is opened by clicking on the glyph positioned at right of each property value and has only one option – Reset (for resetting the property to a default value). You can customize this menu by modifying the xamPropertyGrid’s OptionsMenu property and provide a new instance of type ContextMenu
. The DataContext of the custom ContextMenu will be automatically set to the PropertyGridPropertyItem associated with the current property.
The following code example demonstrates how to define an options menu with the default "Reset" option and also two more options for displaying current property name and current property value.
In XAML:
<ig:XamPropertyGrid x:Name="xamPropertyGrid1">
<ig:XamPropertyGrid.OptionsMenu>
<ContextMenu>
<MenuItem Header="Custom Reset Value">
<ig:Commanding.Command>
<igPrim:PropertyGridPropertyItemCommandSource
EventName="Click"
CommandType="ResetPropertyValue"
ParameterBinding="{Binding}" />
</ig:Commanding.Command>
</MenuItem>
<MenuItem Header="Obtain Property Name" Click="MenuItemName_Click" />
<MenuItem Header="Obtain Property Value" Click="MenuItemValue_Click" />
</ContextMenu>
</ig:XamPropertyGrid.OptionsMenu>
</ig:XamPropertyGrid>
In C#:
private void MenuItemName_Click(object sender, RoutedEventArgs e)
{
MenuItem mi = sender as MenuItem;
PropertyGridPropertyItem pgpi = mi.DataContext as PropertyGridPropertyItem;
MessageBox.Show(pgpi.PropertyName, "Property Name");
}
private void MenuItemValue_Click(object sender, RoutedEventArgs e)
{
MenuItem mi = sender as MenuItem;
PropertyGridPropertyItem pgpi = mi.DataContext as PropertyGridPropertyItem;
MessageBox.Show(pgpi.Value.ToString(), "Property Value");
}
In Visual Basic:
Private Sub MenuItemName_Click(sender As Object, e As RoutedEventArgs)
Dim mi As MenuItem = TryCast(sender, MenuItem)
Dim pgpi As PropertyGridPropertyItem = TryCast(mi.DataContext, PropertyGridPropertyItem)
MessageBox.Show(pgpi.PropertyName, "Property Name")
End Sub
Private Sub MenuItemValue_Click(sender As Object, e As RoutedEventArgs)
Dim mi As MenuItem = TryCast(sender, MenuItem)
Dim pgpi As PropertyGridPropertyItem = TryCast(mi.DataContext, PropertyGridPropertyItem)
MessageBox.Show(pgpi.Value.ToString(), "Property Value")
End Sub
The following topics provide additional information related to this topic.