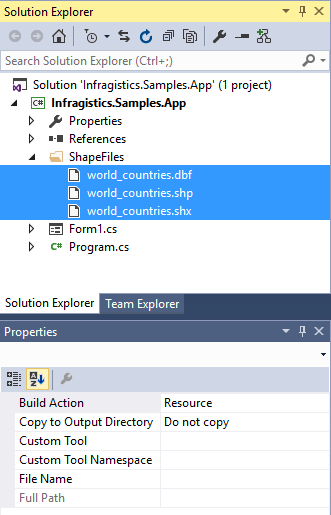
This topic provides information on how to load shape files and bind geo-spatial data to the UltraGeographicMap™ control.
The following table lists the topics required as a prerequisite to understanding this topic.
This topic contains the following sections:
Adding shape files to an application works the same way as adding typically non-executable data files such as image, audio, and video files. As a developer, you have a number of methods for deploying shape files with your application. These methods are explained in the following MSDN topic:
All shape files should be placed in the same folder in your application.
The following image show a sample structure of Windows Forms application project with shape files added as Resource files:
In the UltraGeographicMap control, the ShapefileConverter class loads geo-spatial data from shape files and converts it to a collection of ShapefileRecord objects.
The following table explains properties of the ShapefileConverter class for loading shape files.
Both of the source properties for shape files are of Uri type. This means that shape files can be embedded resources in the application assembly and on the internet (via http). Refer to the previous section for more information on this process. The rules for resolving Uri objects are equivalent to any standard Uri property, for example the BitmapImage.UriSource property.
When both source properties are set to non-null values, then the ShapefileConverter object’s ImportAsync method is invoked which in return performs fetching and reading the shape files and finally doing the conversion. After this operation is complete, the ShapefileConverter is populated with ShapefileRecord objects and the ImportCompleted event is raised in order to notify about completed process of loading and converting geo-spatial data from shape files.
The following code creates an instance of the ShapefileConverter object for loading a shape file that contains locations of major cities in the world. It also demonstrates how to handle the ImportCompleted event as a prerequisite for binding data to the UltraGeographicMap control.
In Visual Basic:
Imports Infragistics.Win.DataVisualization
Private WithEvents shapefileConverter As New ShapefileConverter()
shapefileConverter.ShapefileSource = New Uri("ShapeFiles/world_countries.shp", UriKind.RelativeOrAbsolute)
shapefileConverter.DatabaseSource = New Uri("ShapeFiles/world_countries.dbf", UriKind.RelativeOrAbsolute)
Private Sub OnShapefileConverterImportCompleted(sender As Object, e As System.ComponentModel.AsyncCompletedEventArgs) Handles shapefileConverter.ImportCompleted
' TODO: bind shapefileConverter to the UltraGeographicMap control
End Sub
In C#:
using Infragistics.Win.DataVisualization;
ShapefileConverter shapefileConverter = new ShapefileConverter();
shapefileConverter.ImportCompleted += OnShapefileImportCompleted;
shapefileConverter.ShapefileSource = new Uri("ShapeFiles/world_countries.shp", UriKind.RelativeOrAbsolute);
shapefileConverter.DatabaseSource = new Uri("ShapeFiles/world_countries.dbf", UriKind.RelativeOrAbsolute);
private void OnShapefileImportCompleted(object sender, System.ComponentModel.AsyncCompletedEventArgs e)
{
// TODO: bind ShapefileConverter to the UltraGeographicMap control
}
In the UltraGeographicMap control, Geographic Series are used for displaying geo-spatial data that is loaded from shape files. Refer to the Using Geographic Series topic for overview of supported Geographic Series by the UltraGeographicMap control. All types of Geographic Series have an DataSource property which can be bound to any object that implements the interface (for example: List, Collection, Queue, Stack). The ShapefileConverter is another example of Enumerable object because it implements a collection of ShapefileRecord objects.
The ShapefileRecord class provides properties for storing geo-spatial data, listed in the following table.
This data structure is suitable for use in most Geographic Series as long as appropriate data columns are mapped to them.
The following topics explain in detail binding and data requirements for each type of Geographic Series:
The following image is a preview of the UltraGeographicMap control with GeographicShapeSeries bound to shape files of the world.
This code example assumes that shape files were loaded using the ShapefileConverter as it is explained in the Adding Shape Files to Application section of this topic.
The following code binds GeographicShapeSeries in the UltraGeographicMap control to the ShapefileConverter and maps the Points property of all ShapefileRecord objects.
In Visual Basic:
Private Sub OnShapefileConverterImportCompleted(sender As Object, e As System.ComponentModel.AsyncCompletedEventArgs) Handles shapeFileConverter.ImportCompleted
Me.GeoMap.Series[0].DataSource = shapefileConverter
Me.GeoMap.Series[0].ShapeMemberPath = “Points”
End Sub
In C#:
private void OnShapefileConverterImportCompleted(object sender, System.ComponentModel.AsyncCompletedEventArgs e)
{
this.GeoMap.Series[0].DataSource = shapefileConverter;
this.GeoMap.Series[0].ShapeMemberPath = “Points”;
}
The following topics provide additional information related to this topic.