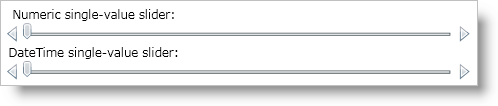
The xamSlider™ controls have tick marks that help your end users distinguish values on the control. The type of tick marks you use will depend on the type of slider you are using. For example, if you are using a numeric slider, i.e., xamNumericSlider™ or xamNumericRangeSlider™, you must use SliderTickMarks objects. On the other hand, if you are using a DateTime slider, i.e., xamDateTimeSlider™ or xamDateTimeRangeSlider™, you must use the DateTimeSliderTickMarks objects. The two types of tick marks are not interchangeable. However, they both share these commonly used properties:
In addition to the properties already mentioned, the DateTimeSliderTickMarks object also exposes a FrequencyType property that you can set to a FrequencyType enum value. The xamDateTimeSlider and xamDateTimeRangeSlider controls will use the TickMarksFrequency property in conjunction with the FrequencyType property to arrange their tick marks. You can think of the TickMarksFrequency property as a scalar value and the FrequencyType property as the unit of measure. For example, if your values range from 1/1/2009 to 12/31/2009 and you set the TickMarksFrequency property to 3, you need to set the FrequencyType property to determine whether tick marks should be displayed every 3 days, months, years, etc.
The following example code demonstrates adding and setting tick mark properties.
In XAML:
<StackPanel> <!--single value numeric slider with tick marks every 20th interval--> <TextBlock Text=" Numeric single-value slider:" /> <ig:XamNumericSlider Name="xamNumericSlider1" MinValue="0" MaxValue="100"> <ig:XamNumericSlider .TickMarks> <ig:SliderTickMarks TickMarksFrequency="20" UseFrequency="True" /> </ig:XamNumericSlider .TickMarks> </ig:XamNumericSlider > <!--single value DateTime slider with tick marks every 2 months--> <TextBlock Text="DateTime single-value slider:" /> <ig:XamDateTimeSlider Name="xamDateTimeSlider1" MinValue="1/1/2009" MaxValue="12/31/2009"> <ig:XamDateTimeSlider.TickMarks> <ig:DateTimeSliderTickMarks TickMarksFrequency="2" FrequencyType="Months" UseFrequency="True" /> </ig:XamDateTimeSlider.TickMarks> </ig:XamDateTimeSlider> </StackPanel>
In Visual Basic:
Imports Infragistics.Controls.Interactions Imports Infragistics ... 'Tick marks for a single value numeric slider Dim numericTickMarks As New SliderTickMarks With {.TickMarksFrequency = 20, .UseFrequency = True} Me.xamNumericSlider1.TickMarks.Add(numericTickMarks) 'Tick marks for a single value DateTime slider Dim dateTickMarks As New DateTimeSliderTickMarks With {.TickMarksFrequency = 2, .FrequencyType = FrequencyType.Months, .UseFrequency = True} Me.xamDateTimeSlider1.TickMarks.Add(dateTickMarks) ...
In C#:
using Infragistics.Controls.Interactions; using Infragistics; ... //Tick marks for a single value numeric slider SliderTickMarks numericTickMarks = new SliderTickMarks { TickMarksFrequency = 20, UseFrequency = true }; this.xamNumericSlider1.TickMarks.Add(numericTickMarks); //Tick marks for a single value DateTime slider DateTimeSliderTickMarks dateTickMarks = new DateTimeSliderTickMarks { TickMarksFrequency = 2, FrequencyType = FrequencyType.Months, UseFrequency = true }; this.xamDateTimeSlider1.TickMarks.Add(dateTickMarks); ...